一些基础知识,长期更新
一些有关java的基础学习笔记,之前一直在书上记,后面想想还是不太方便
变量
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| public class bianliang1 { public static void main(String[] args){ int a = 10; System.out.println(a); int b = 20; System.out.println(a + b); a = 50; System.out.println(a + b); int e = 100, f = 200, g = 300; System.out.println(e); System.out.println(f); System.out.println(g); int r; r = 900; System.out.println(r); } }
10 30 70 100 200 300
|
变量注意事项:
- 只能存一个值
- 变量名不允许重复定义(可以修改值)
- 一条语句可以定义多个变量
- 变量在使用之前一定要赋值
- 变量的作用域范围
计算机的存储规则
- Text 文本
- image 图片
- Sound 声音
计算机中任意数据都是以二进制形式存储的。
1 2 3 4 5 6 7 8 9 10 11
|
public class bianliang1 { public static void main(String[] args){ System.out.println(017); System.out.println(0b1111111); System.out.println(0xa7); } }
|
数据类型
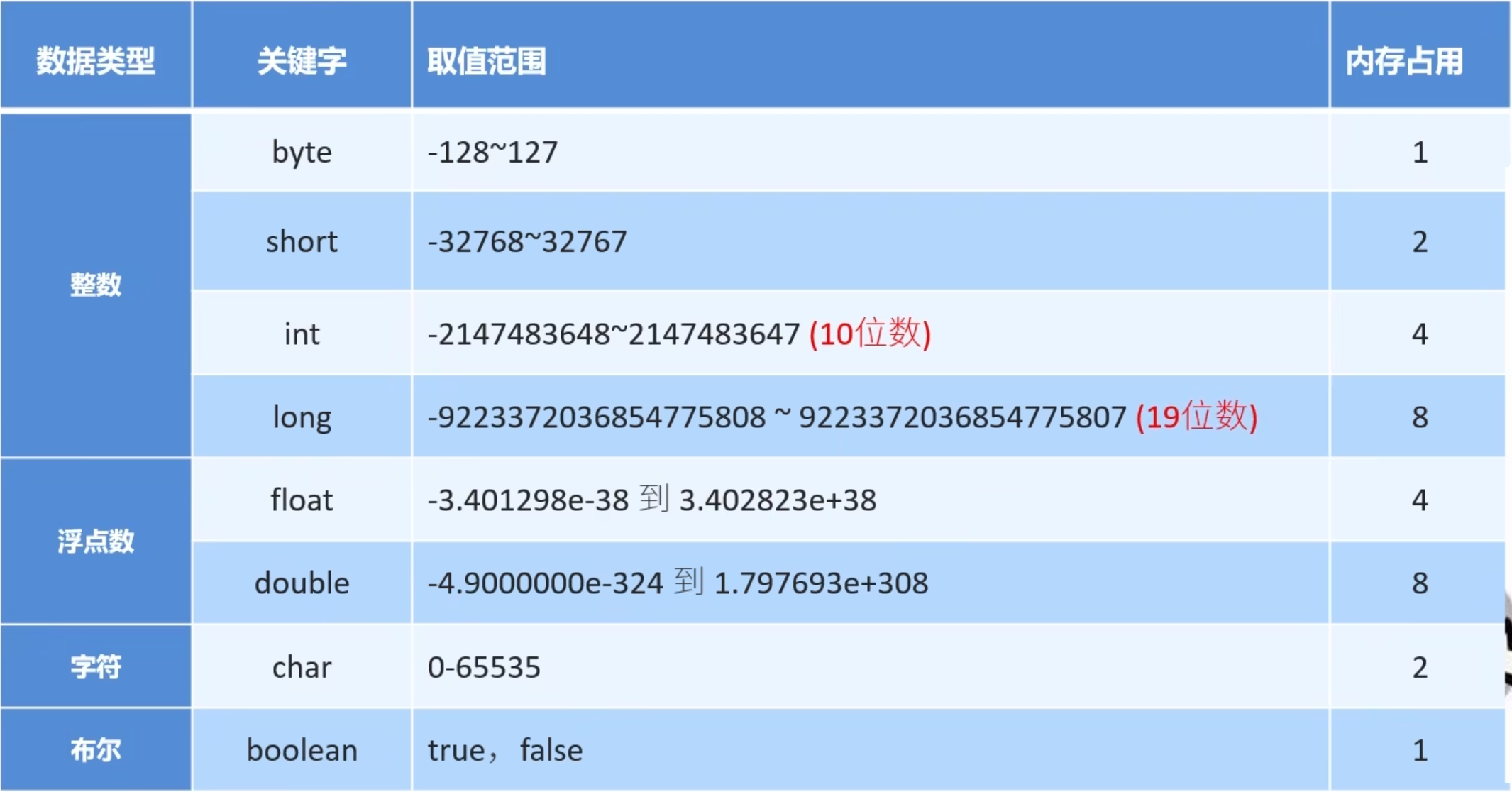
1 2 3 4 5 6 7 8 9 10 11 12
| public class bianliang1 { public static void main(String[] args){ byte a = 127; short b = 20; int c = 30; long d = 40L; float e = 1.1F; double f = 2.2; char g = '任'; boolean k = false; } }
|
标识符
就是给类、方法、变量等起的名字。
- 可以由数字、字母、下划线(_)和美元符($)组成。
- 不能以数字开头
- 不能是关键字
- 区分大小写
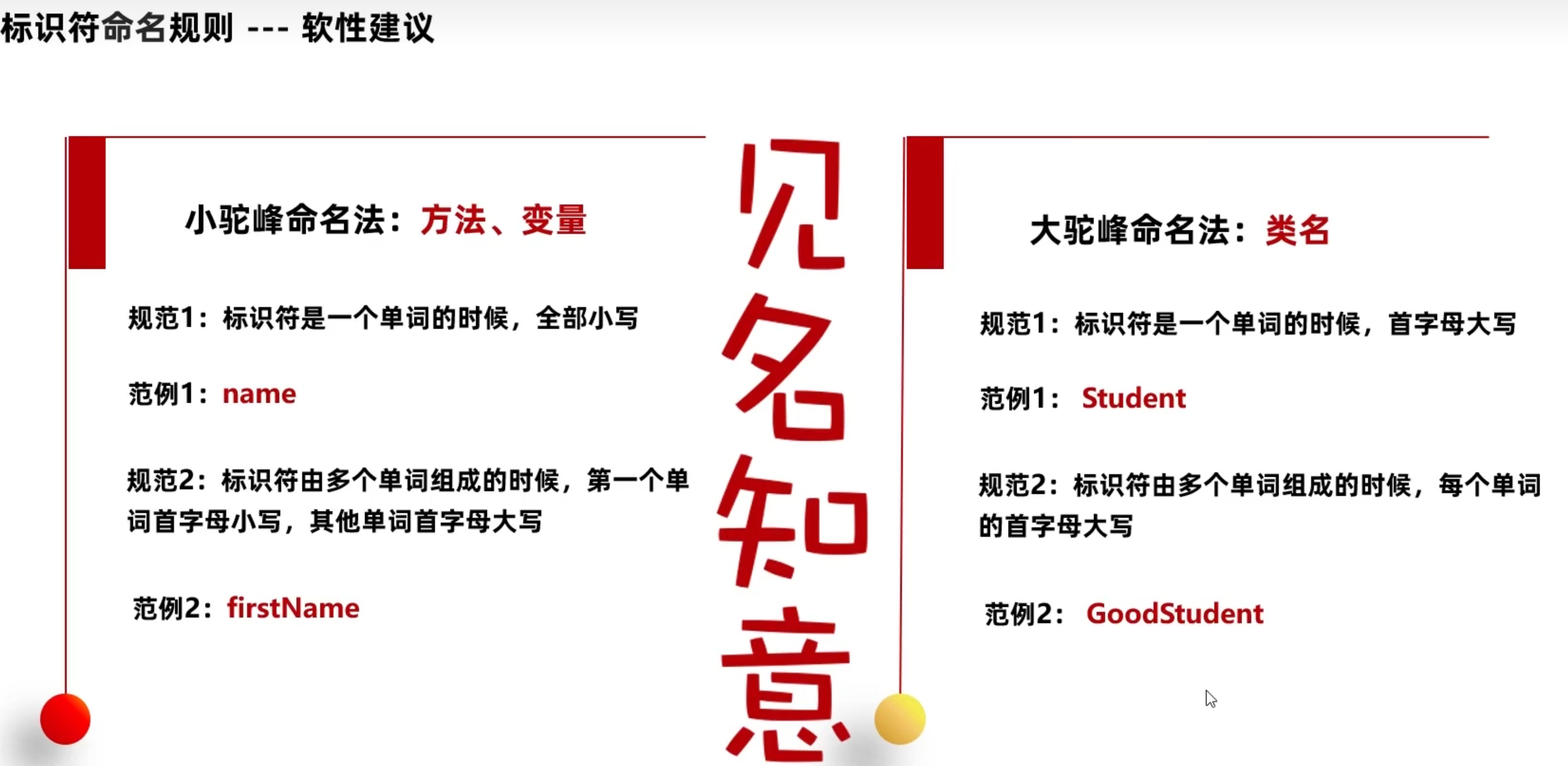
键盘录入
Scanner这个类可以接收键盘输入
1 2 3
| import java.util.Scanner; Scanner sc = new Scanner(System.in); int i = sc.nextInt();
|
比如:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| import java.util.Scanner;
public class bianliang1 { public static void main(String[] args){
Scanner sc = new Scanner(System.in); System.out.println("请输入一个整数:");
int i = sc.nextInt();
System.out.println(i); } }
|
键盘输入两个整数并求和:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| import java.util.Scanner;
public class bianliang1 { public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入一个整数i:");
int num1 = sc.nextInt();
System.out.println("请再输入一个整数j:");
int num2 = sc.nextInt();
int Jieguo = num1 + num2;
System.out.println("两数之和为:" + Jieguo); } }
|
运算符
算数运算符:加减乘除取模/取余(%)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| public class bianliang1 { public static void main(String[] args){
System.out.println(1 + 1);
System.out.println(2 - 1);
System.out.println(2 * 2);
System.out.println(9 / 3);
System.out.println(10 / 3);
System.out.println(10.0 / 3); System.out.println(9 % 2);
System.out.println(1.1 + 1.01);
} }
|
练习:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| public class bianliang1 { public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入一个三位数num1:");
int num1 = sc.nextInt();
int baiwei = (num1 - (num1 % 100)) / 100;
int shiwei = ((num1 - baiwei * 100) - (num1 - baiwei *100) % 10) / 10;
int gewei = (num1 - baiwei *100 - shiwei * 10);
System.out.println("百位是:" + baiwei);
System.out.println("十位是:" + shiwei);
System.out.println("个位是:" + gewei); } }
|
算数运算符
类型转换:
- 隐式转换(自动类型提升):取值范围小的数值转换为取值范围大的。
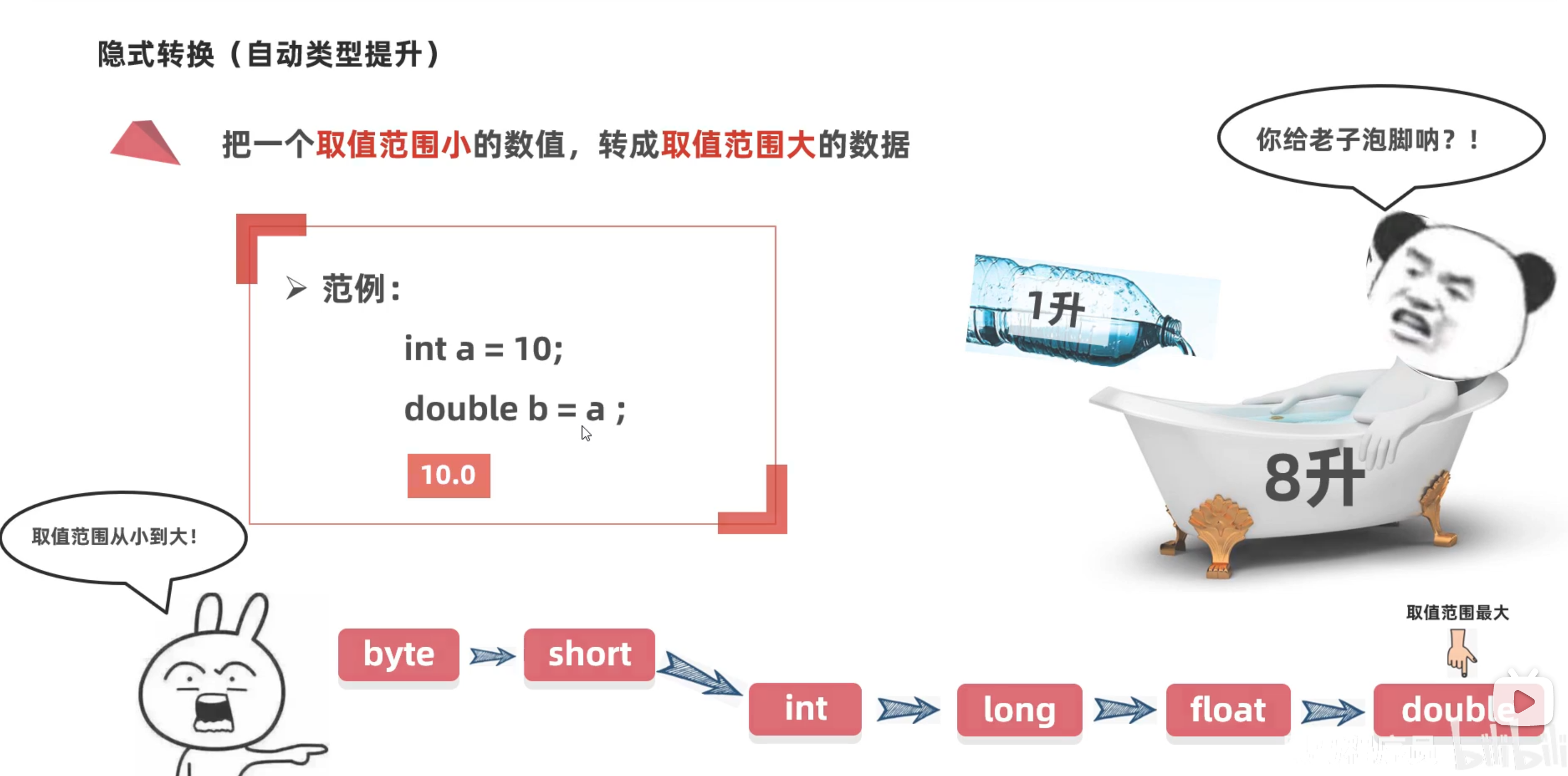
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| public class bianliang1 { public static void main(String[] args){ int a = 10;
double b = 11.1;
数据类型? c = a + b;
System.out.println(c);
} }
public class bianliang1 { public static void main(String[] args){ byte a = 10;
byte b = 11.1;
数据类型? c = a + b;
System.out.println(c);
} }
|
取值范围:byte < short < int < long < float < double
数据类型不一样时不能进行计算,转换成一样的才能计算。
两个转换规则。
格式:目标数据类型 变量名 = (目标数据类型)被强转的数据;
1 2
| double a = 12.3; int b = (int) a;
|
字符串 “+” 操作:
当 “ + ” 操作中出现字符串时,这个 “ + ”是字符串连接符,而不是算数运算符。会将前后的数据进行拼接,并产生一个新的字符串。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| public class bianliang1 { public static void main(String[] args){ System.out.println(3.7 + "abc");
System.out.println("abc" + true);
System.out.println('中' + "abc" + true);
int age = 18;
System.out.println("我的年龄是:" + age + "岁");
System.out.println("我的年龄是:" + "age" + "岁");
System.out.println(1 + 2 + "abc" + 2 + 1);
} }
|
字符的+
操作
当字符+字符
或字符+数字
时,会把字符通过ASCII
码表查询到对应的数字再进行计算。
1 2
| System.out.println(1 + 'a' ); System.out.println('a' + "abc" );
|
自增自减运算符++,--
- 单独使用:
++
和--
无论是放在变量前面还是后面,单独写一行结果时一样的
1 2 3 4
| int a = 10; ++a; sout(a)
|
1 2 3 4 5
| int a = 10; int b = a++;
int a = 10; int b = ++a;
|
练习:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| public class bianliang1 { public static void main(String[] args){ int x = 10;
int y = x++;
int z = ++x;
System.out.println("x: " + x);
System.out.println("y: " + y);
System.out.println("z: " + z);
} }
|
赋值运算符
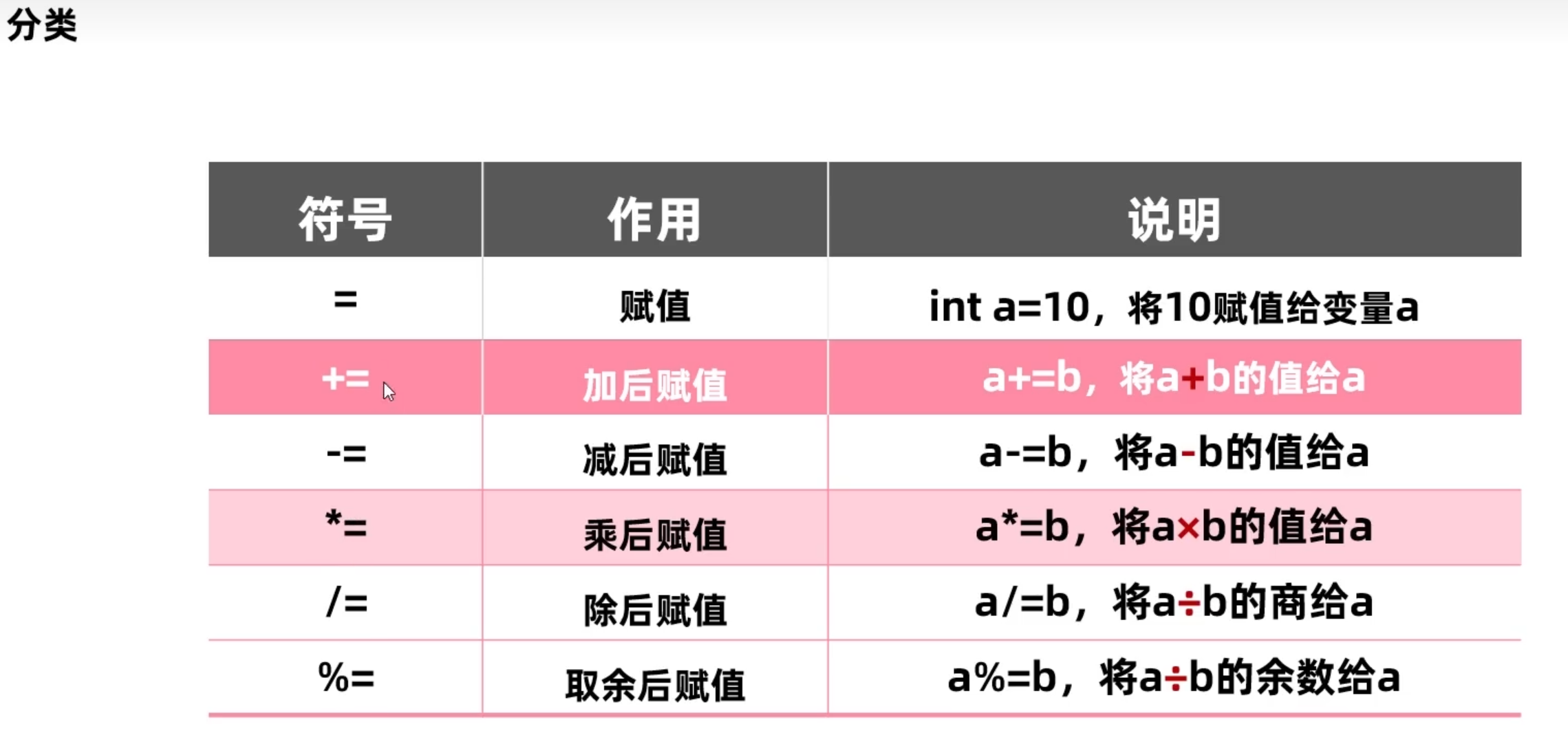
比如:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| public class bianliang1 { public static void main(String[] args){ int a = 1;
int b = 2;
a+=b;
System.out.println("a: " + a);
a-=b;
System.out.println("a: " + a);
a*=b;
System.out.println("a: " + a);
a/=b;
System.out.println("a: " + a);
a%=b;
System.out.println("a: " + a); short s = 1; } }
|
关系运算符
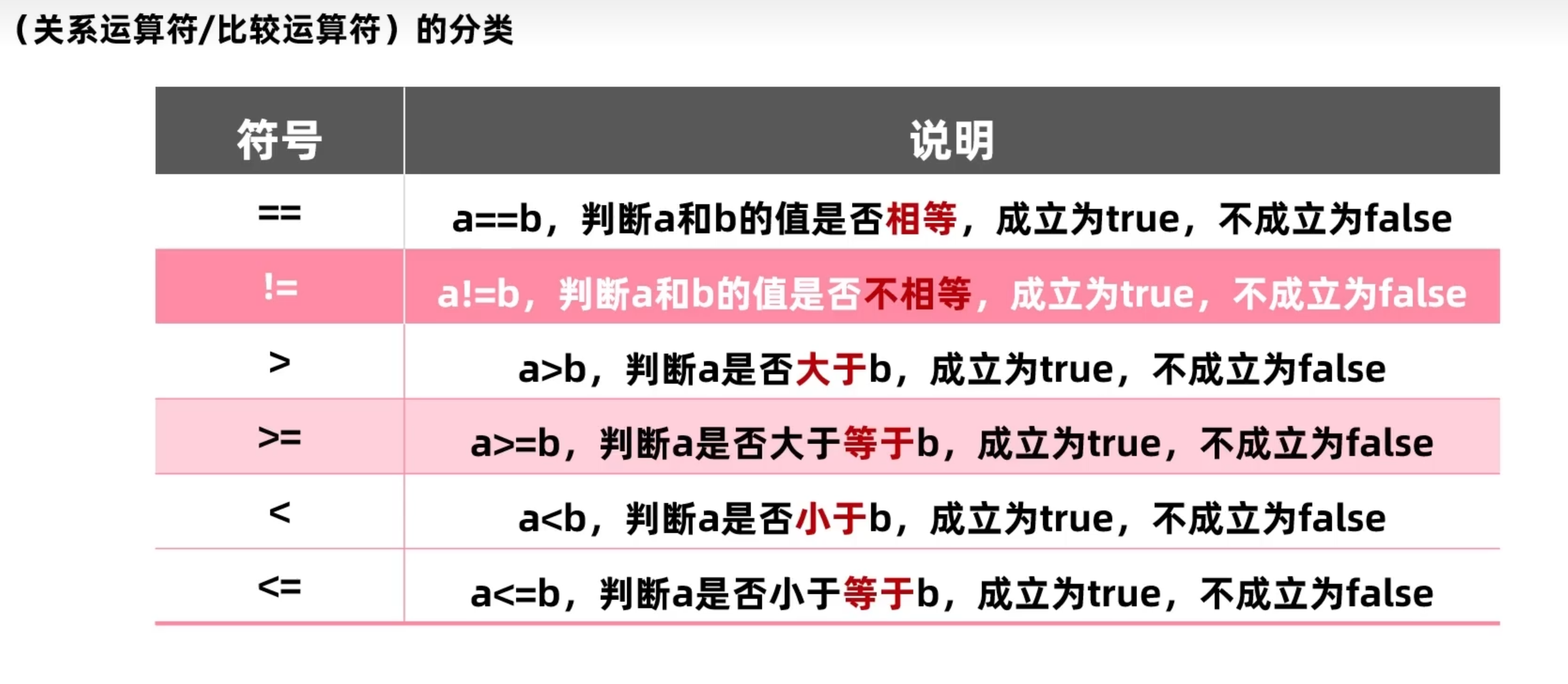
注意:关系运算符的结果都是boolean类型,即要么true要么false。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| public class bianliang1 { public static void main(String[] args){ int a = 1;
int b = 2;
int c = 1;
System.out.println(a == b);
System.out.println(a == c);
} }
|
练习:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| import java.util.Scanner; public class bianliang1 { public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入对方分数(0-10):");
int num1 = sc.nextInt();
System.out.println("请输入你的分数(0-10):");
int num2 = sc.nextInt();
boolean a = num2 >= num1;
System.out.println(a);
} }
|
逻辑运算符
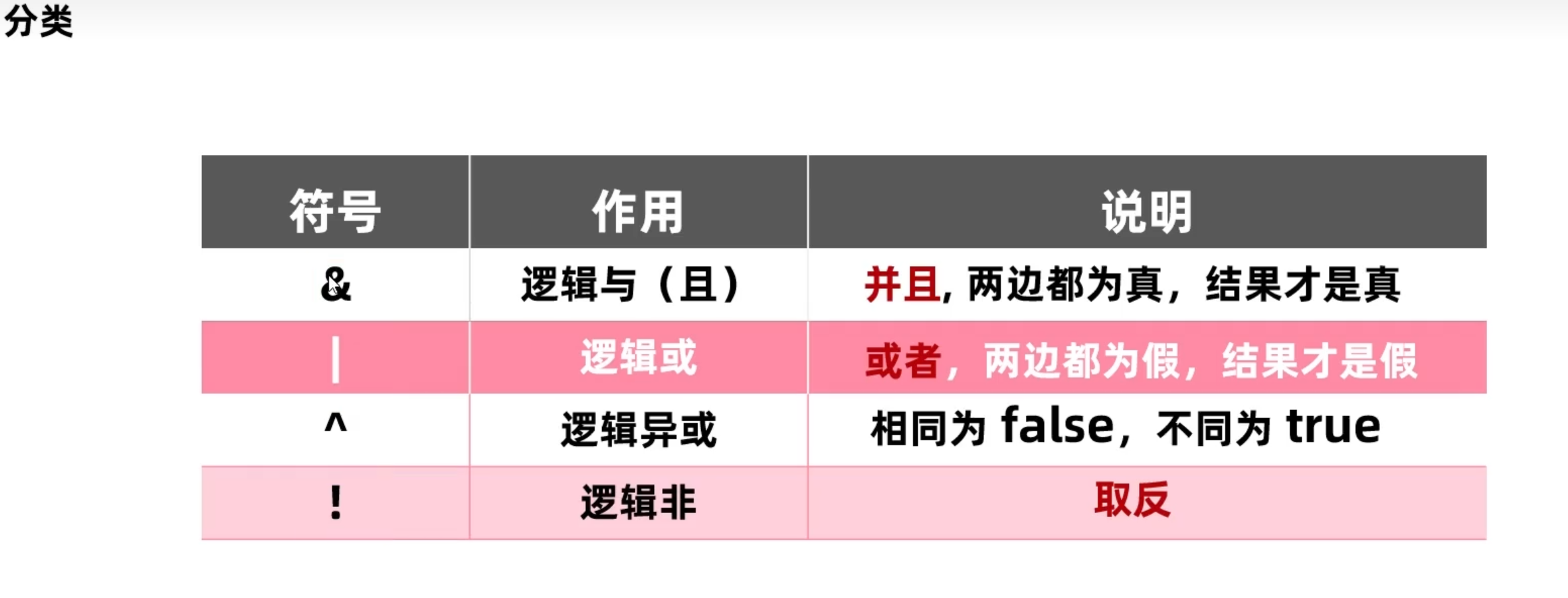
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| public class bianliang1 { public static void main(String[] args){ System.out.println(true & true);
System.out.println(true & false);
System.out.println(true | false);
System.out.println(false | false);
System.out.println(false ^ true);
System.out.println(!false);
} }
|
短路逻辑运算符
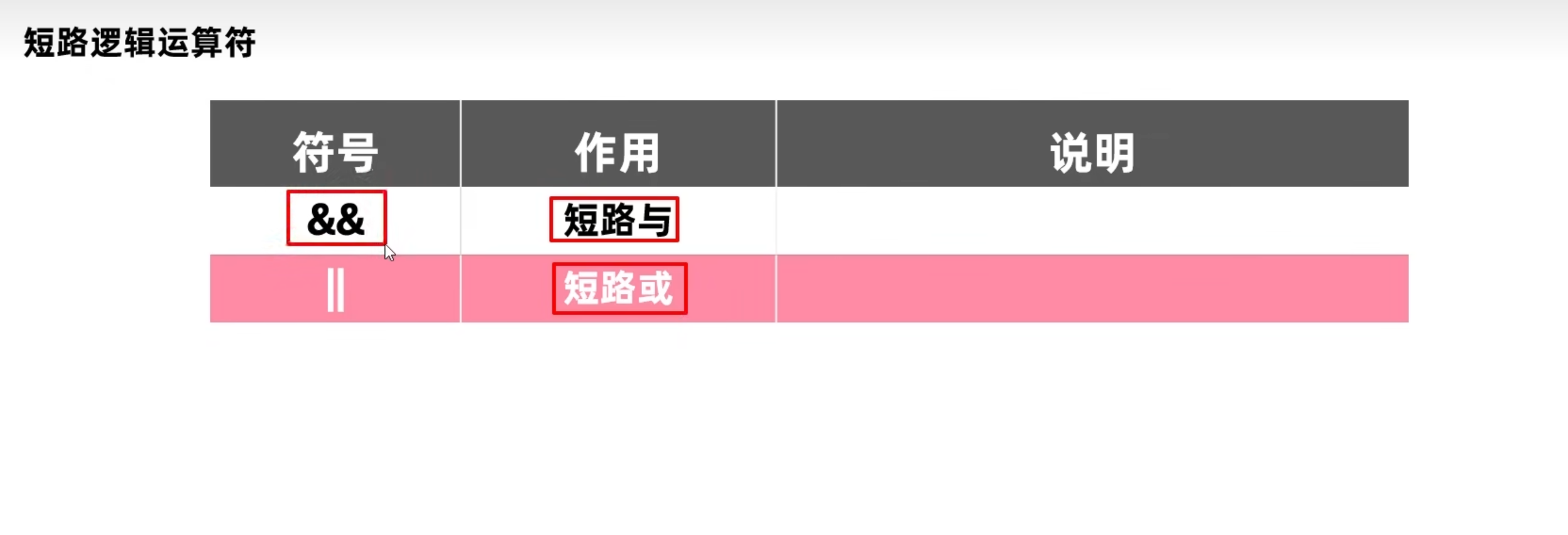
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| ```
```java public class bianliang1 { public static void main(String[] args){ System.out.println(true && true);
System.out.println(true && false);
System.out.println(true || false);
System.out.println(false || false);
int a = 10;
int b = 20;
boolean result = ++a < 5 && ++b < 5;
System.out.println(a);
System.out.println(b);
boolean result2 = ++a < 5 & ++b < 5;
System.out.println(a);
System.out.println(b); } }
|
练习:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| import java.util.Scanner; public class bianliang1 { public static void main(String[] args){ Scanner sc = new Scanner(System.in);
System.out.println("请输入一个数num1:");
int num1 = sc.nextInt();
System.out.println("请输入一个数num2:");
int num2 = sc.nextInt();
boolean result1 = num1 == 6 || num2 == 6;
System.out.println(result1);
boolean result2 = (num1 + num2) % 6 == 0;
System.out.println(result2);
} }
|
分支结构if
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67
| import java.util.Scanner; public class bianliang1 { public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入你口袋里的钱:");
int money = sc.nextInt();
if (money > 100) { System.out.println("111"); }else { System.out.println("222"); } } }
import java.util.Scanner; public class bianliang1 { public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入你的号码:");
int number = sc.nextInt();
if (number <=100 && number >=0){ if (number % 2==1) { System.out.println("请坐在左边"); }else { System.out.println("请坐在右边"); } }else { System.out.println("不符合规定!"); }
} }
import java.util.Scanner; public class bianliang1 { public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入小明的成绩:");
int number = sc.nextInt();
if (number <=100 && number >=0){ if (number >= 95) { System.out.println("一等奖"); }else if (number <= 94 && number >= 90){ System.out.println("二等奖"); }else if (number <= 89 && number >=85){ System.out.println("三等奖"); }else { System.out.println("无奖"); } }else { System.out.println("请输入合理的成绩!"); }
} }
|
分支结构switch
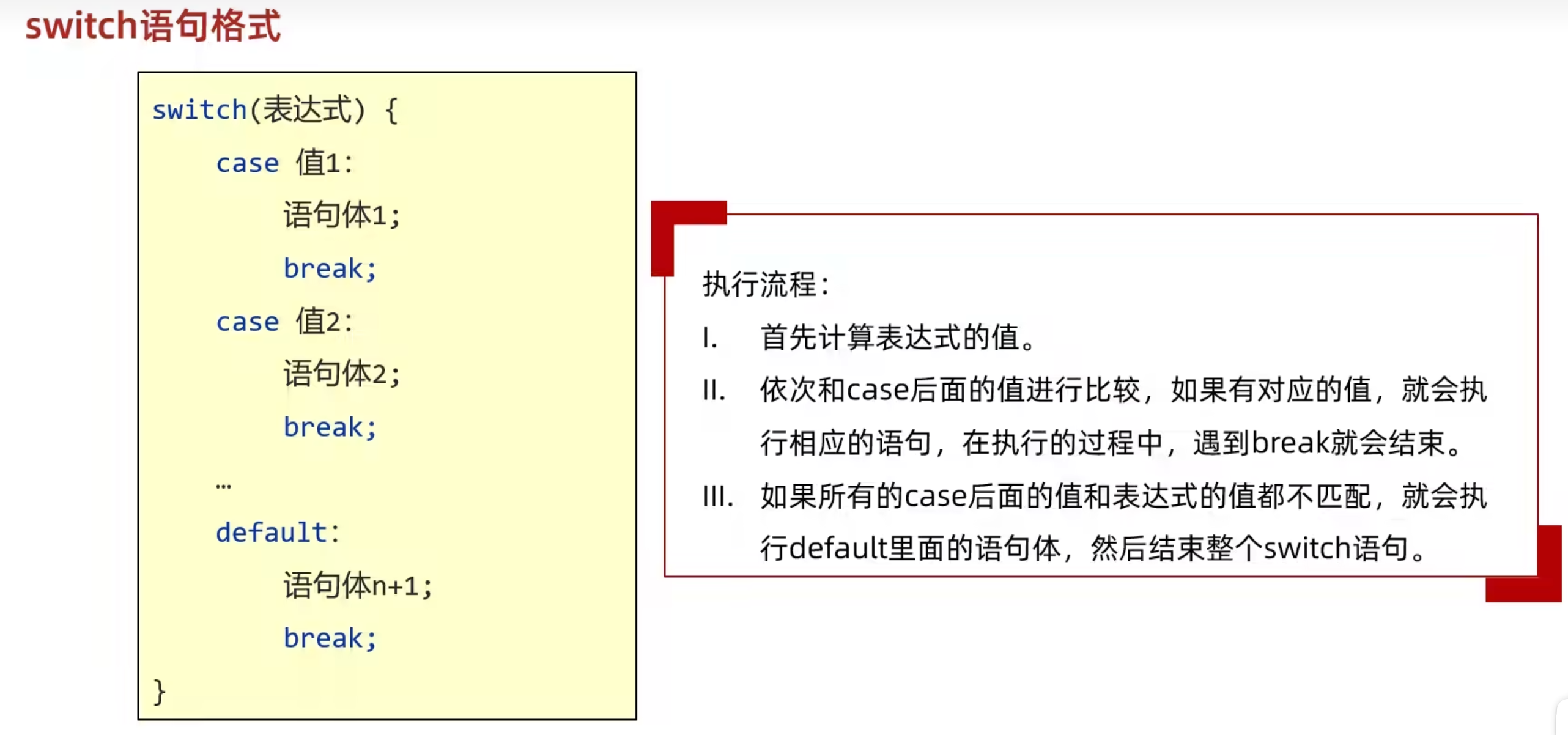
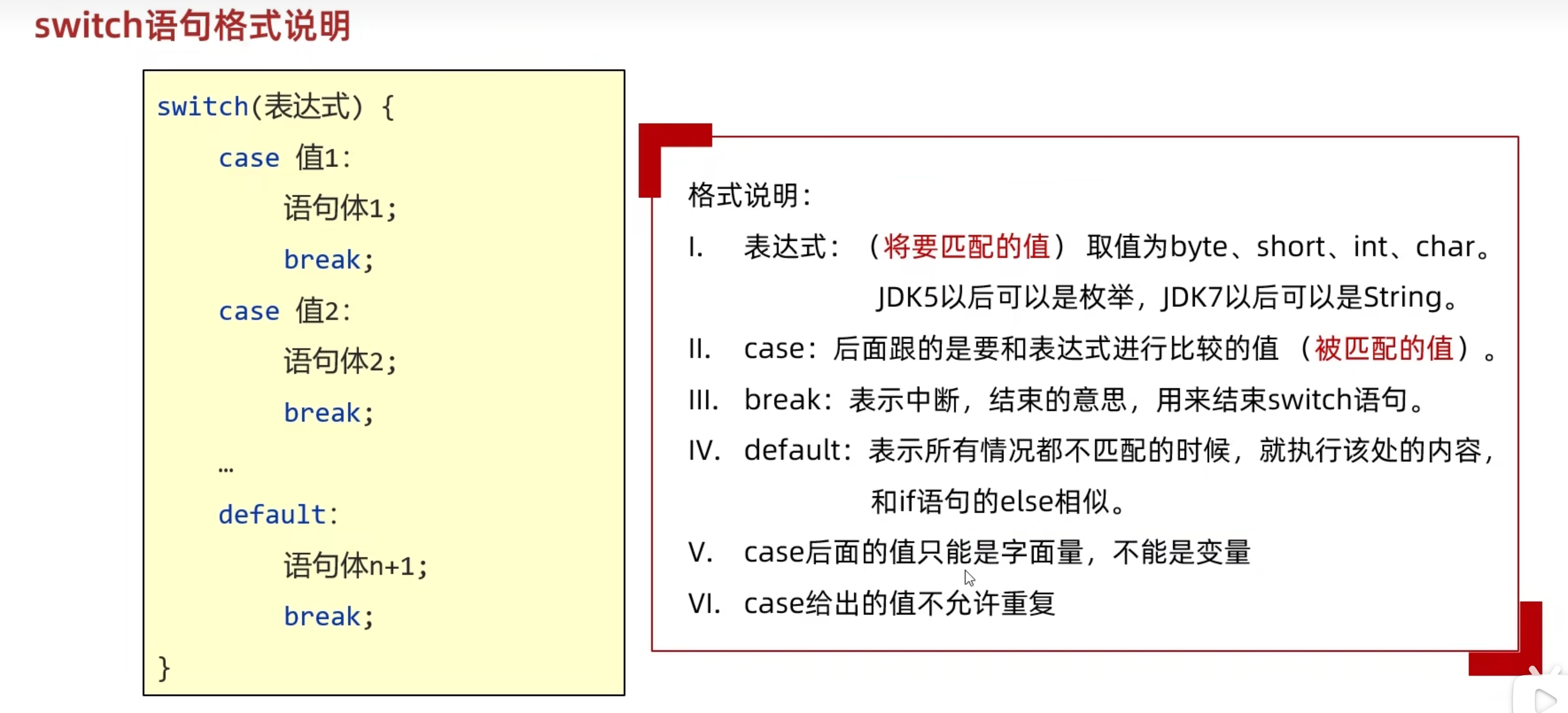
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| import java.util.Scanner; public class bianliang1 { public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入面条种类:");
String noodles = sc.next();
switch (noodles){ case "热干面": System.out.println("reganmian"); break; case "兰州拉面": System.out.println("lanzhoulamian"); break; case "炸酱面": System.out.println("zhajiangmian"); break; default: System.out.println("fangbianmian"); break; } } }
|
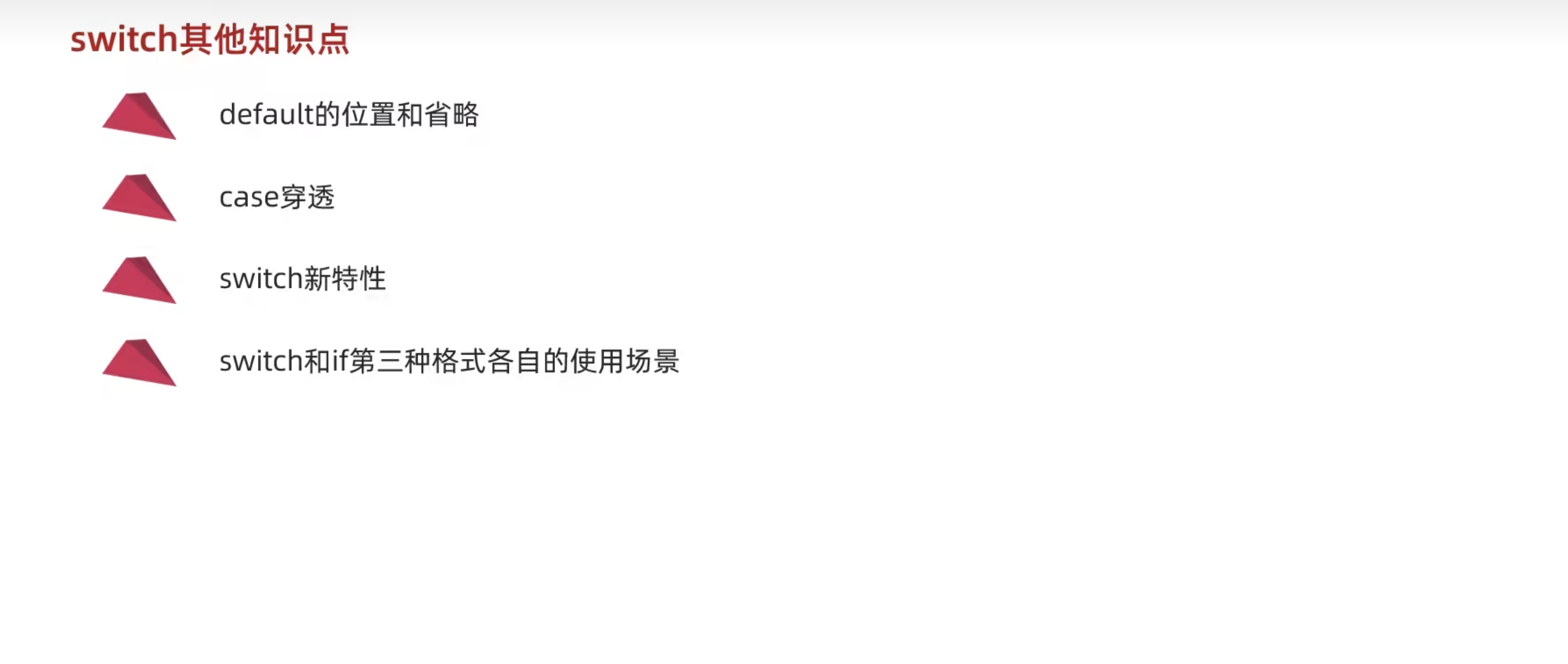
default的位置和省略
case穿透
发现break
会结束整个switch
语句。如果没有发现break
,那么程序会执行下一个case
的语句体,一直遇到break
或大括号为止,比如:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| import java.util.Scanner; public class bianliang1 { public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入今天是星期几:");
String today = sc.next();
switch (today){ case "星期一": System.out.println("reganmian"); case "星期二": System.out.println("lanzhoulamian"); case "星期三": System.out.println("zhajiangmian"); default: System.out.println("fangbianmian"); break; } } }
|
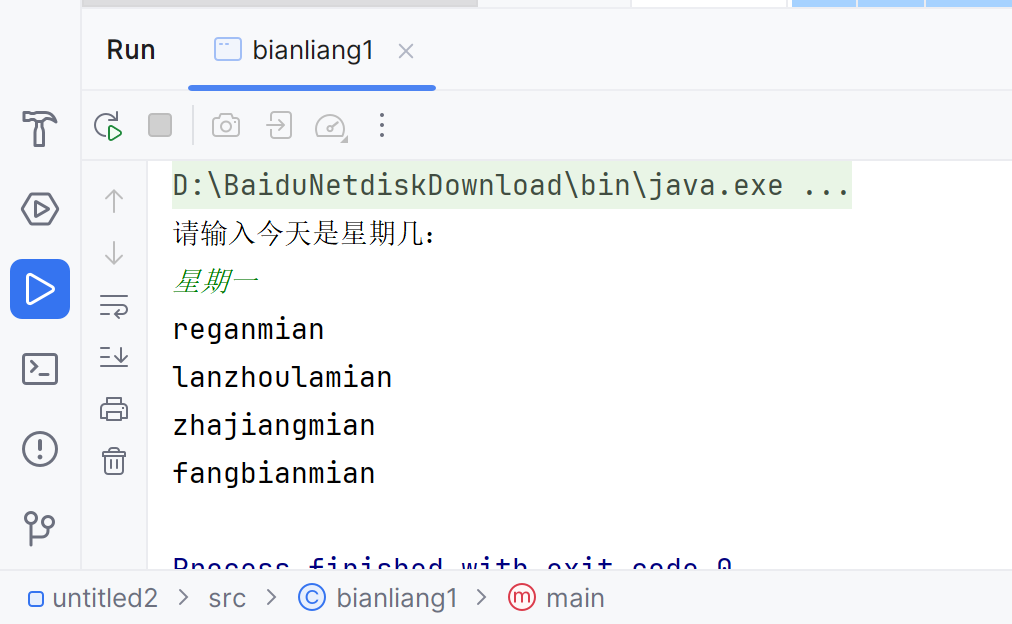
switch的新特性
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| import java.util.Scanner; public class bianliang1 { public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入今天是星期几:");
String today = sc.next();
switch (today){ case "星期一" -> { System.out.println("今天是星期一"); } case "星期二" -> { System.out.println("今天是星期二"); }
case "星期三" -> {
System.out.println("今天是星期三"); } default -> { System.out.println("haha "); }
} } }
|
swicth与if的区别
- switch更多的去一一列举有限个数据
- if更多的去进行范围判断
case穿透例题:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| import java.util.Scanner; public class bianliang1 { public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入今天是星期几:");
String today = sc.next();
switch (today){ case "星期一":
case "星期二":
case "星期三":
case "星期四":
case "星期五": System.out.println("工作日"); break; case "星期六":
case "星期日": System.out.println("休息日"); break;
} } }
|
当然也可以进一步简化(我的版本有点低就只放图了):
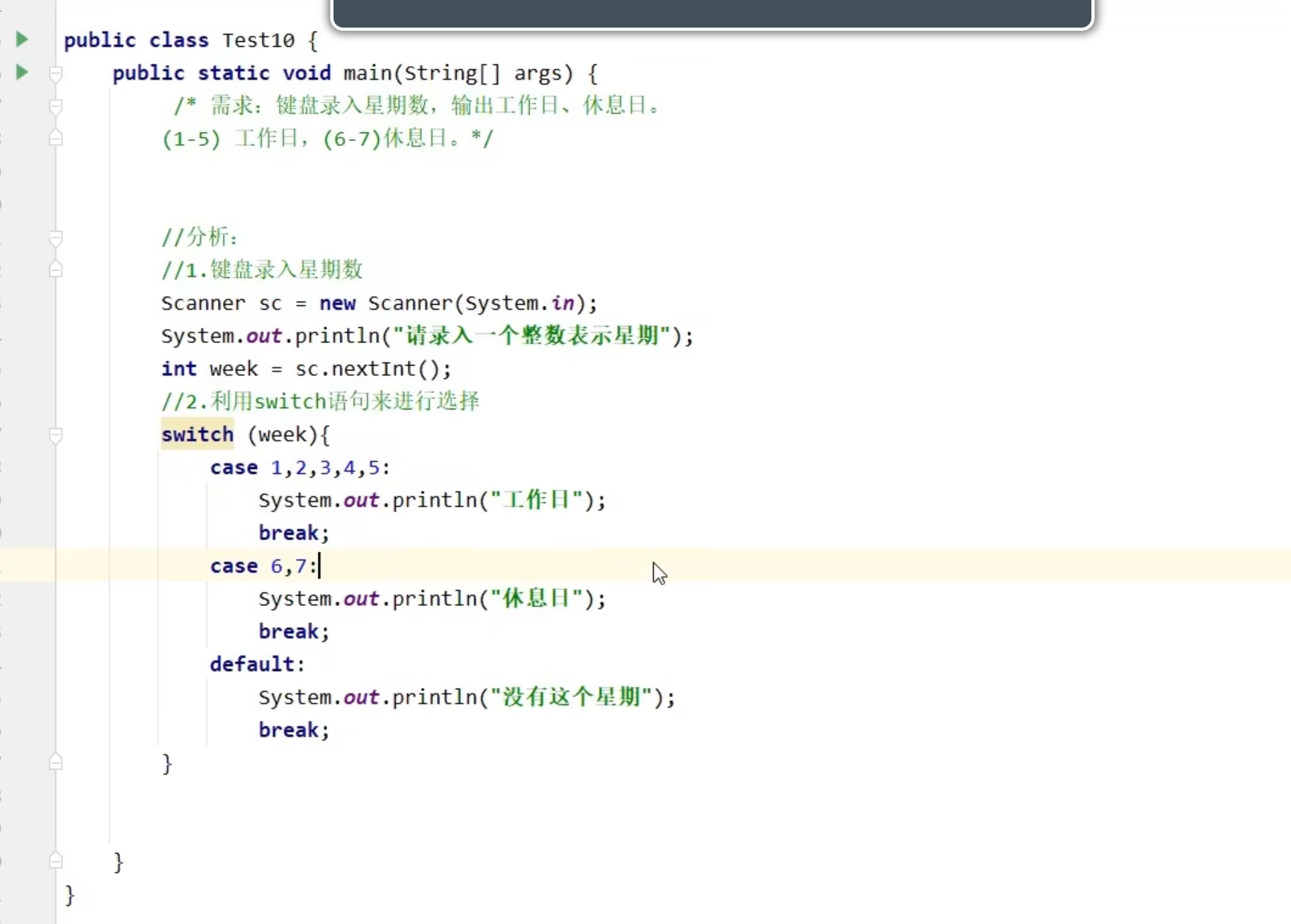
循环结构
for:
1 2 3 4 5 6 7 8 9 10
| public class bianliang1 { public static void main(String[] args){
for (int i = 1; i < 10; i++){ System.out.println("you should know me"); }
} }
|
练习:求1-100中所有偶数之和
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| public class bianliang1 { public static void main(String[] args){ int sum = 0;
for (int i = 1; i <= 100; i++){
if (i % 2 ==0){
sum = sum +i;
} } System.out.println(sum);
} }
|
练习:键盘录入两个数字,表示一个范围。统计这个范围中既能被三整除又能被五整除的数字有对多少个
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| import java.util.Scanner; public class bianliang1 { public static void main(String[] args){
int i;
int sum = 0;
Scanner sc = new Scanner(System.in);
System.out.println("请输入范围较小的数字Num1:");
int num1 = sc.nextInt();
System.out.println("请输入范围较大的数字Num2:");
int num2 = sc.nextInt();
for (i = num1; i <= num2; i++){ if (i % 3 ==0 && i % 5 ==0){ sum = sum +1; } }
System.out.println("在" + num1 + "到" + num2 + "这个范围中,能同时被3和5整除的数字的数量为:"+sum);
} }
|
while:
1 2 3 4 5 6 7 8 9 10 11
| public class bianliang1 { public static void main(String[] args) { int i = 1; while (i <= 100) { System.out.println(i); i++;
} } }
|
for和while的对比:
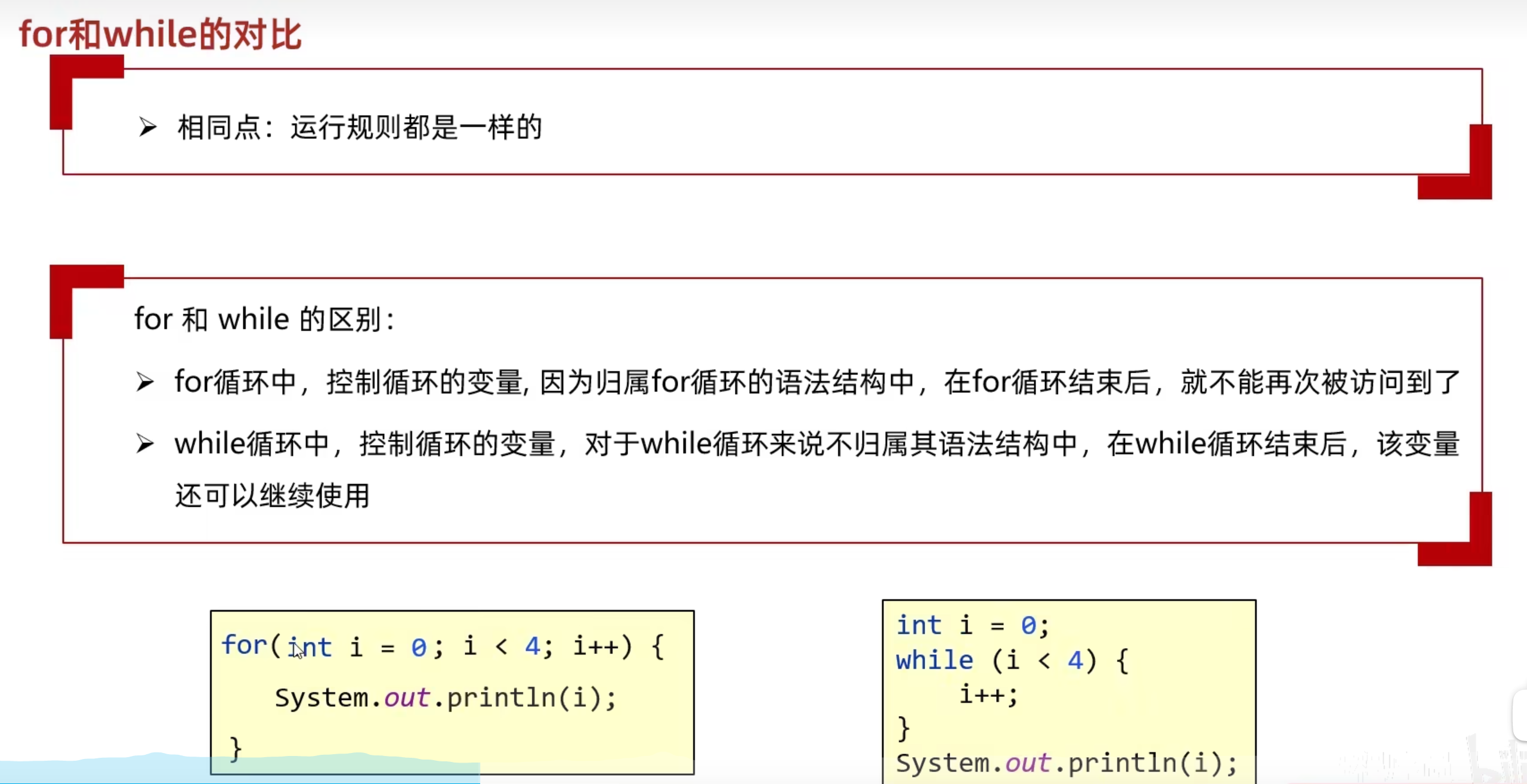
比如对于这段代码:
1 2 3 4 5 6 7 8 9 10 11
| public class bianliang1 { public static void main(String[] args) { int i = 1; while (i <= 10) { System.out.println(i); i++;
} System.out.println(i); } }
|
最后输出的数据会是11
当然可以对for
进行如下改写(i提到外面):
1 2 3 4 5 6 7 8 9 10 11
| public class bianliang1 { public static void main(String[] args) { int i = 1; for ( ; i <= 10;i++) { System.out.println(i);
} System.out.println(i); } }
|
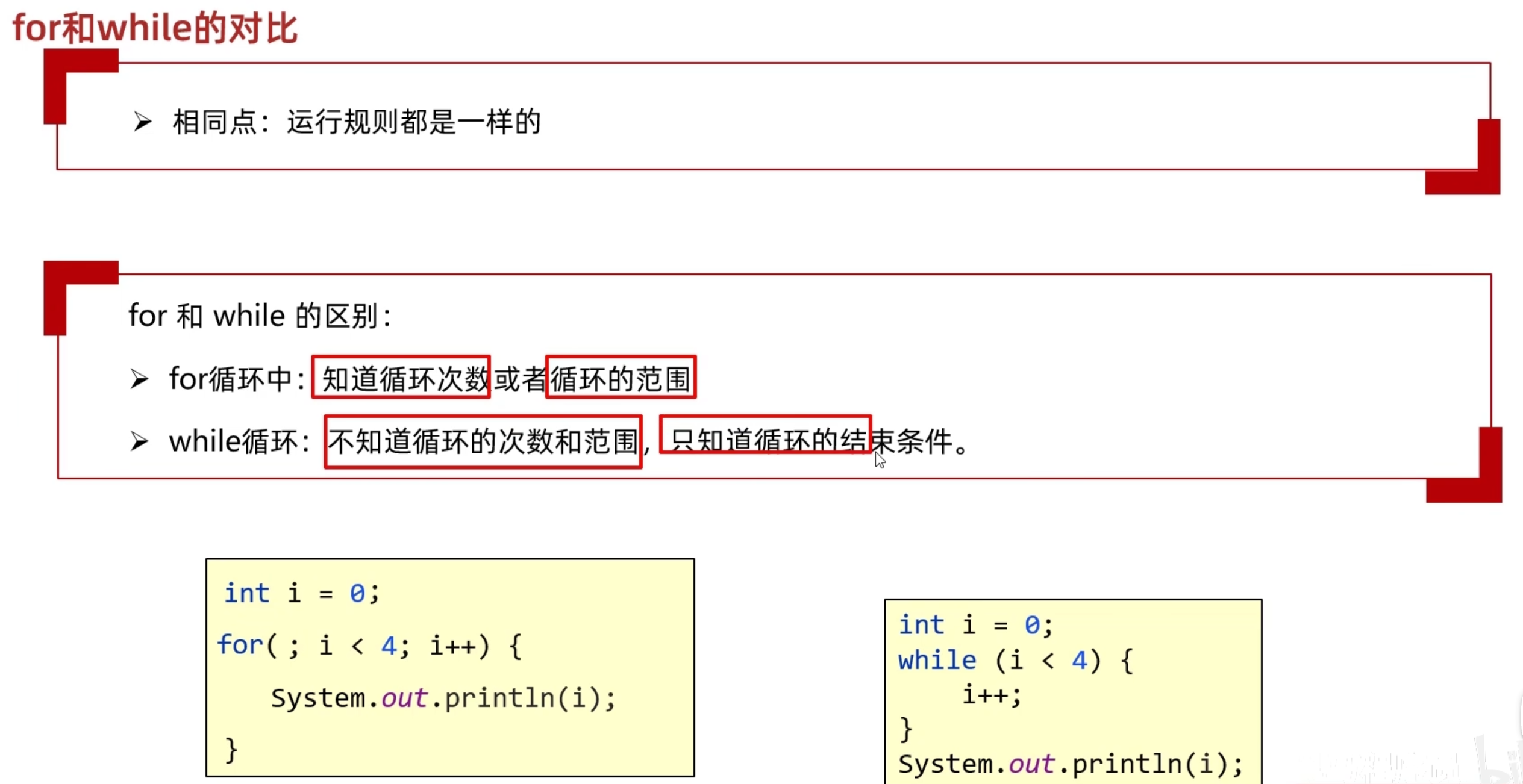
练习:如果想把0.1mm
的纸折叠成8844430mm
,求折叠的次数
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| public class bianliang1 { public static void main(String[] args) { int i = 0;
double hight = 0.1;
while (hight <= 8844430){
hight = hight * 2;
i = i +1; } System.out.println(i); } }
|
判断回文数:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| ```
P48
P54:
### 数组
> 数组指的是一种容器,可以用来存储同种数据类型的多个值

数组的定义

数组初始化方式:
- 静态初始化:

比如:
```java public class bianliang1 { public static void main(String[] args) { int [] theirage = {1, 2, 3, 4, 5}; String [] theirname = {"tom", "jack", "bob", "chris"}; double [] theirhight = {111.1, 222.2, 333.3, 444.4}; } }
|
如何将数组中的数据取出?如果直接sout
数组名,比如:
1 2 3 4 5 6
| public class bianliang1 { public static void main(String[] args) { int [] theirage = {1, 2, 3, 4, 5}; System.out.println(theirage); } }
|
会出现:
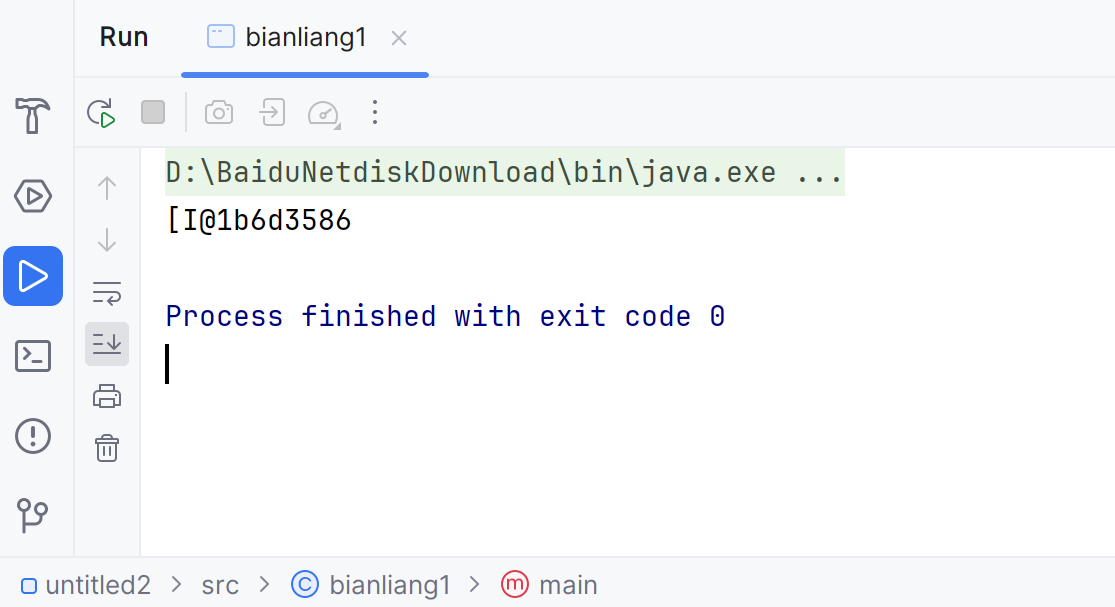
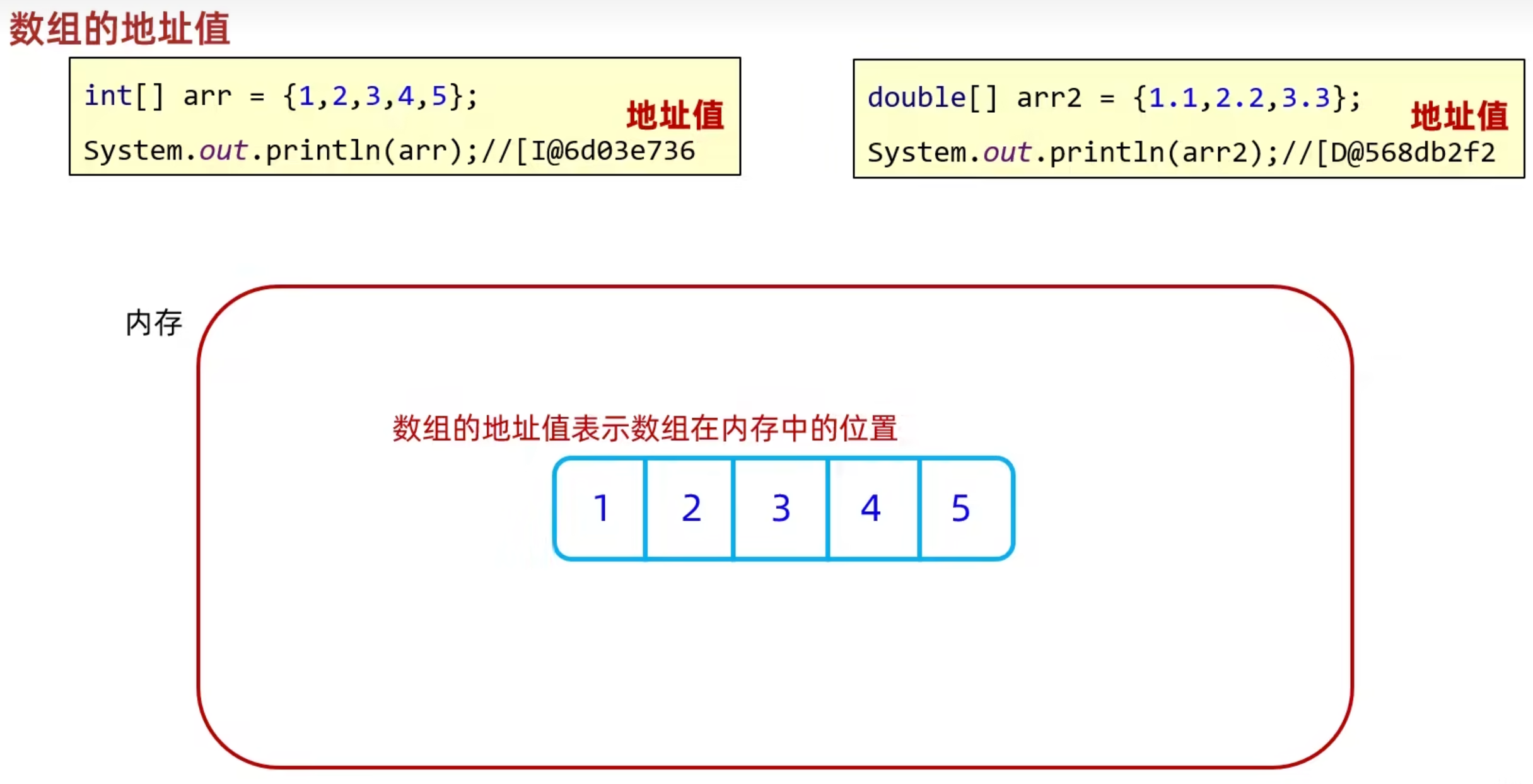
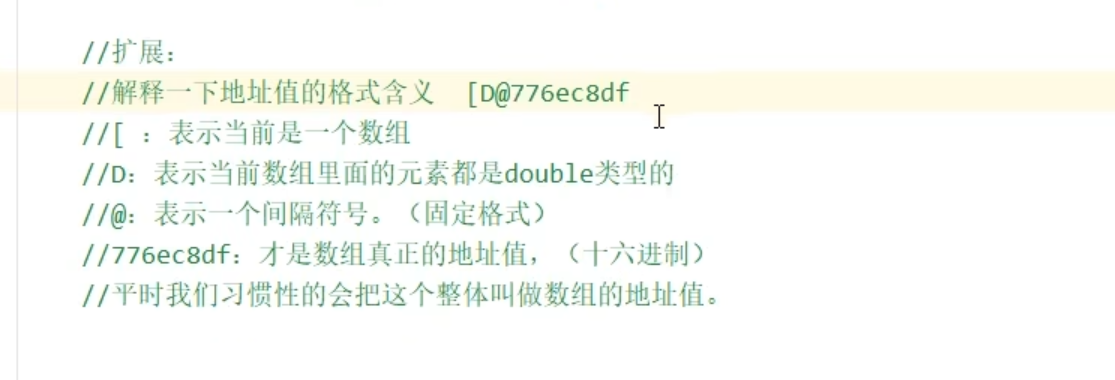
数组元素访问
索引:
- 也叫下标、角标。
- 特点:从0开始,逐个+1增长,连续不间断。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| public class bianliang1 { public static void main(String[] args) {
int [] theirage = {1, 2, 3, 4, 5};
int array1 = theirage[0];
System.out.println(array1);
System.out.println(theirage[0]);
theirage[0] = 100;
System.out.println(theirage[0]);
} }
|
数组遍历
- 将数组中所有的内容取出来,可以对其进行一系列操作:打印,求和,判断等
- 遍历指的是取出数据的过程,不要局限的理解为遍历就是打印
跟python
差不多,还是用for
循环进行遍历:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| public class bianliang1 { public static void main(String[] args) {
int [] theirage = {1, 2, 3, 4, 5, 1, 2, 3, 4, 5};
int arraylength = theirage.length;
System.out.println(arraylength);
int i;
for (i=0; i < arraylength; i++){
System.out.println(theirage[i]);
}
} }
|
当然idea
也提供了快速生成数组的方式,直接数组名.fori
就会自动生成for (i=0; i < arraylength; i++)
这东西
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101
| public class bianliang1 { public static void main(String[] args) {
int [] theirage = {1, 2, 3, 4, 5};
int arraylength = theirage.length;
System.out.println(arraylength);
int sum = 0;
for (int i1 = 0; i1 < theirage.length; i1++) {
System.out.println(theirage[i1]);
sum = sum + theirage[i1];
}
System.out.println("数组元素之和为:" + sum);
} }
public class bianliang1 { public static void main(String[] args) {
int [] theirage = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int arraylength = theirage.length;
System.out.println(arraylength);
int sum = 0;
int i = 0;
for (int i1 = 0; i1 < theirage.length; i1++) {
System.out.println(theirage[i1]);
sum = sum + theirage[i1];
if (theirage[i1] % 3 ==0){
System.out.println("该数字:" + theirage[i1] + "可以被3整除");
i = i +1;
}
}
System.out.println("数组元素之和为:" + sum); System.out.println("该数组中一共有" + i + "个数可被3整除");
} }
public class bianliang1 { public static void main(String[] args) {
int [] theirage = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int arraylength = theirage.length;
System.out.println(arraylength);
int i = 0;
for (int i1 = 0; i1 < theirage.length; i1++) {
System.out.println(theirage[i1]);
if (theirage[i1] % 2 !=0){
int doubleit = theirage[i1] * 2;
theirage[i1] = doubleit;
System.out.println("该数字是奇数,称2后的结果是:" + doubleit);
}else { int chuer = theirage[i1] / 2;
theirage[i1] = chuer;
System.out.println("该数字是偶数,除二后的结果是:" + chuer); }
}
for (int i1 = 0; i1 < theirage.length; i1++) {
System.out.println(theirage[i1]);
} } }
|
数组动态初始化
- 初始化时只指定数组长度,由系统为数组分配初始值。
- 格式:数据类型[] 数组名 = new 数组类型[数组长度];
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| public class bianliang1 { public static void main(String[] args) {
String[] arr = new String[50];
arr[0] = "zhangsan";
arr[1] = "lisi";
System.out.println(arr[0]);
System.out.println(arr[1]);
System.out.println(arr[2]);
int[] arr2 = new int[3];
System.out.println(arr2[0]);
System.out.println(arr2[1]);
System.out.println(arr2[2]);
} }
|
结果:
1 2 3 4 5 6
| zhangsan lisi null 0 0 0
|
静态初始化和动态初始化的区别:
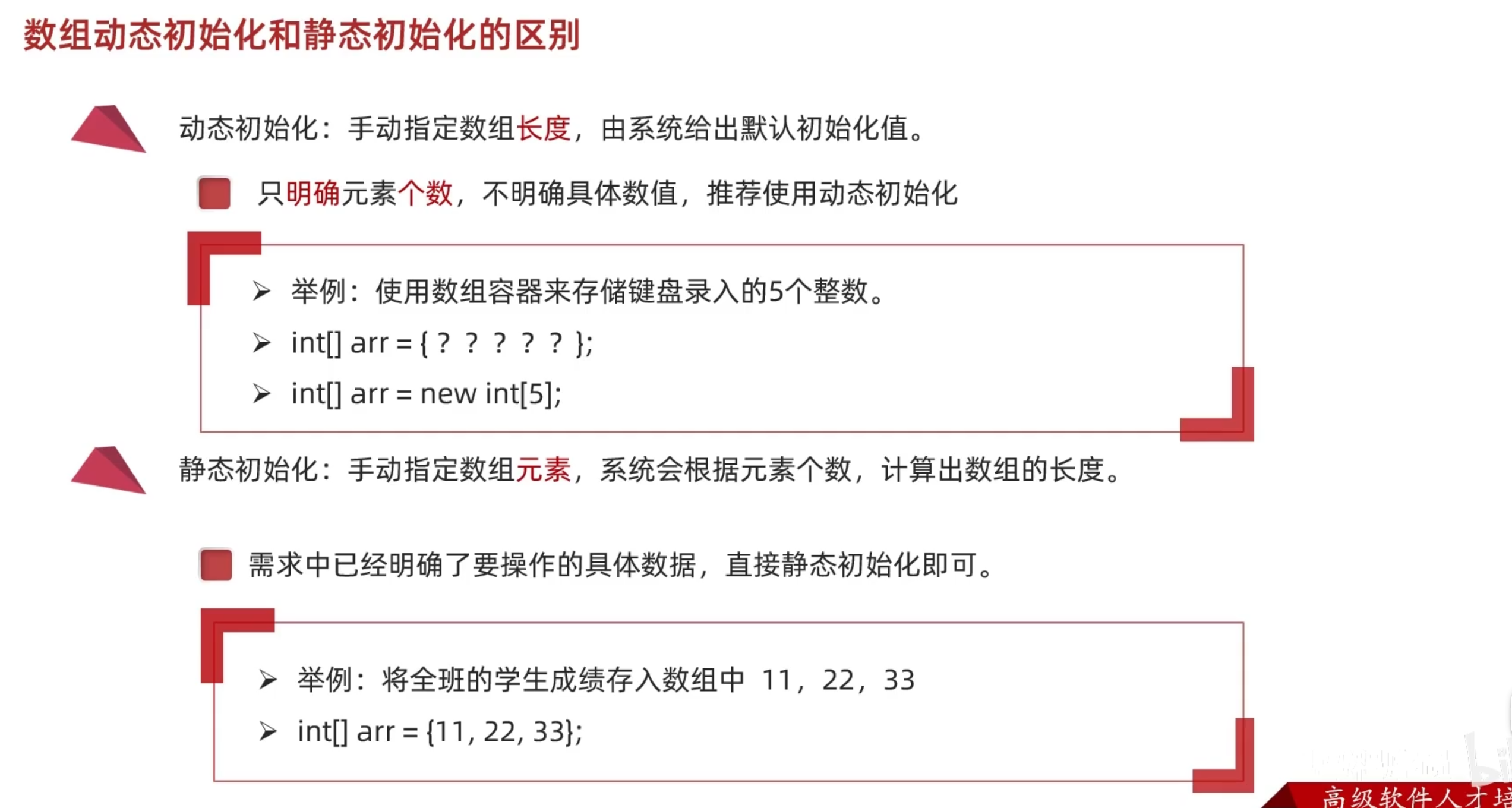
数组常见问题
- 当访问了数组中不存在的索引,就会引发索引越界异常。
数组常见操作
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| public class bianliang1 { public static void main(String[] args) {
int[] arr = {33, 22, 5, 44, 55};
int max = arr[0];
for (int i = 0; i < arr.length; i++) {
if (arr[i] > max){
max = arr[i];
} } System.out.println("数组中的最大值max为:" + max); } }
|
练习:遍历数组求和,求平均数,求有多少个数据比平均值小
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| import java.util.Random;
public class bianliang1 { public static void main(String[] args) { int sum = 0; int num2 = 0; int[] arr = new int[10];
Random r = new Random();
for (int i = 0; i < arr.length; i++) {
int number = r.nextInt(100) + 1;
arr[i] = number;
} int firstnum = arr[0]; for (int i = 0; i < arr.length; i++) { sum = sum + arr[i]; System.out.println("第" + i + "个元素的值为:" + arr[i]); }
System.out.println("数组中这十个元素的和为:" + sum); double pingjunnum = sum / 10; System.out.println("该数组的平均值为:" + pingjunnum); for (int i = 0; i < arr.length; i++) { if (arr[i] > pingjunnum){ num2 = num2 + 1; }
} System.out.println("数组中共有:" + num2 + "个数比平均值大"); } }
|
练习:交换数组中的数据
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| public class bianliang1 { public static void main(String[] args) { int sum = 0; int num2 = 0; int[] arr = {1, 2, 3, 4, 5}; for (int i = 0, j = arr.length - 1; i < j; i++, j--){ int tmp = arr[j];
arr[j] = arr[i];
arr[i] = tmp;
} for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
}
}
import java.util.Random;
public class bianliang1 { public static void main(String[] args) { int[] arr = {1, 2, 3, 4, 5};
for (int i = 0; i < arr.length; i++) { Random r = new Random();
int suiji = r.nextInt(arr.length);
int tmp = arr[i];
arr[i] = arr[suiji];
arr[suiji] = tmp; } for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
} } }
|
Java内存分配
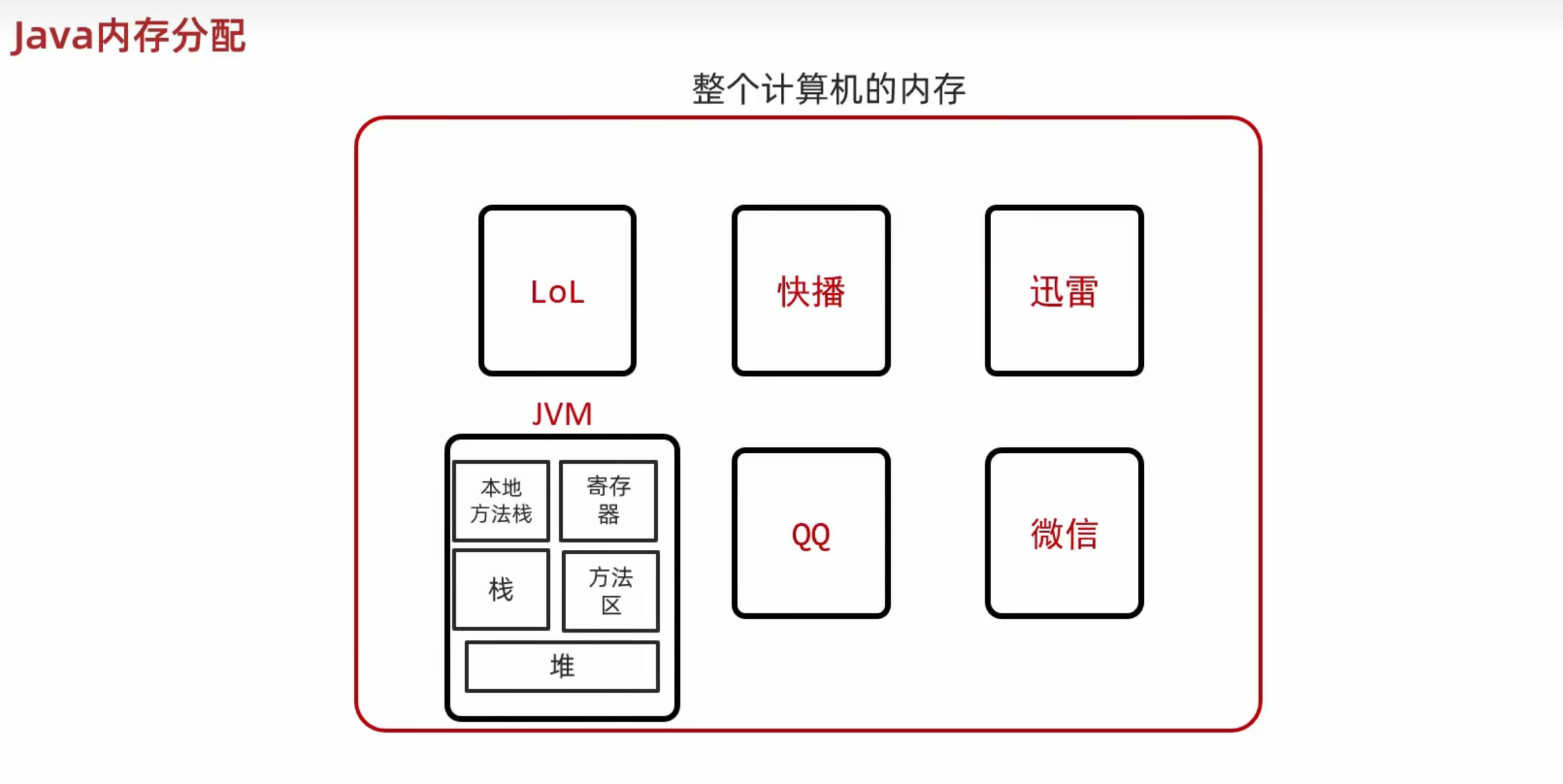
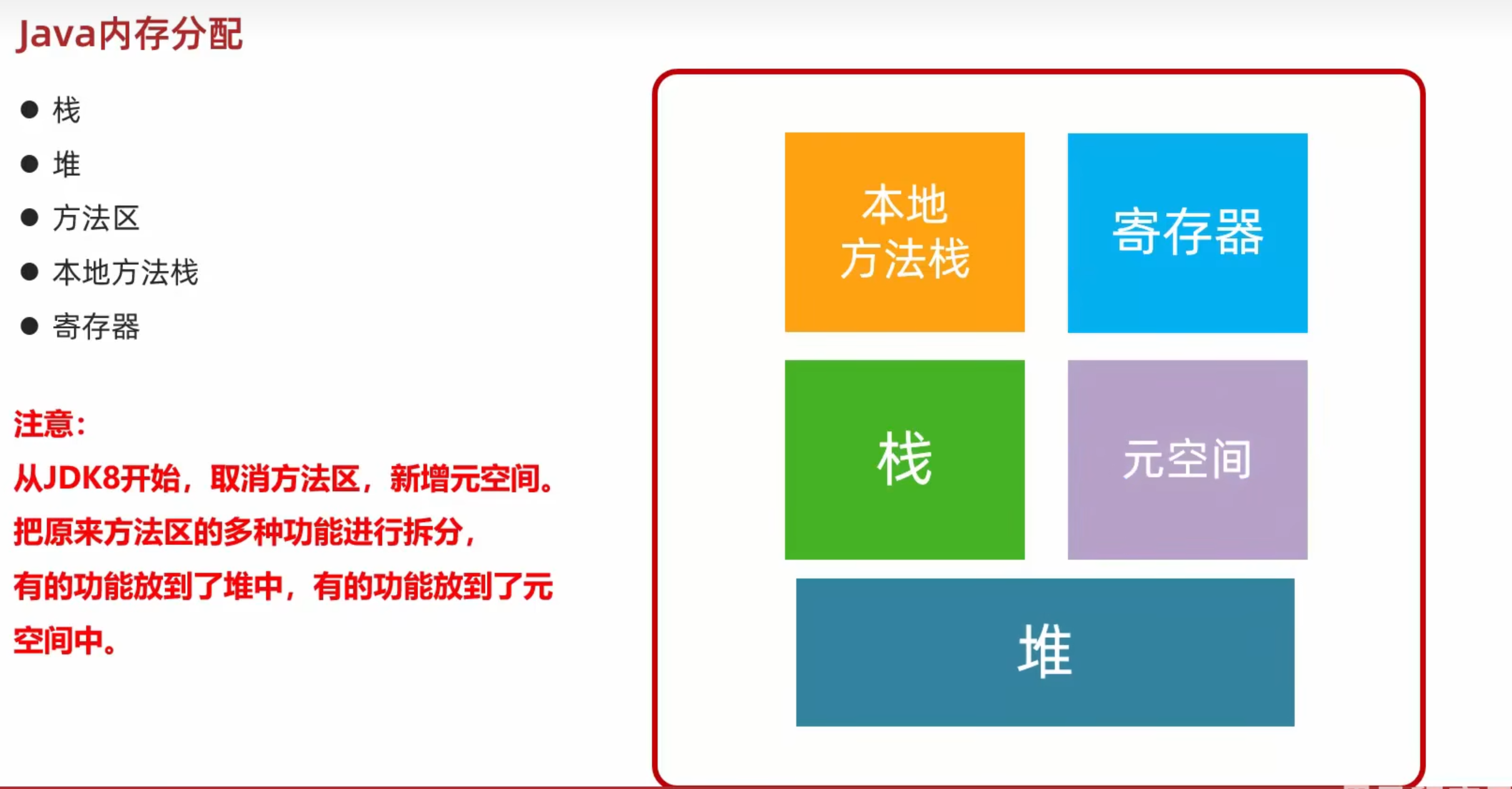
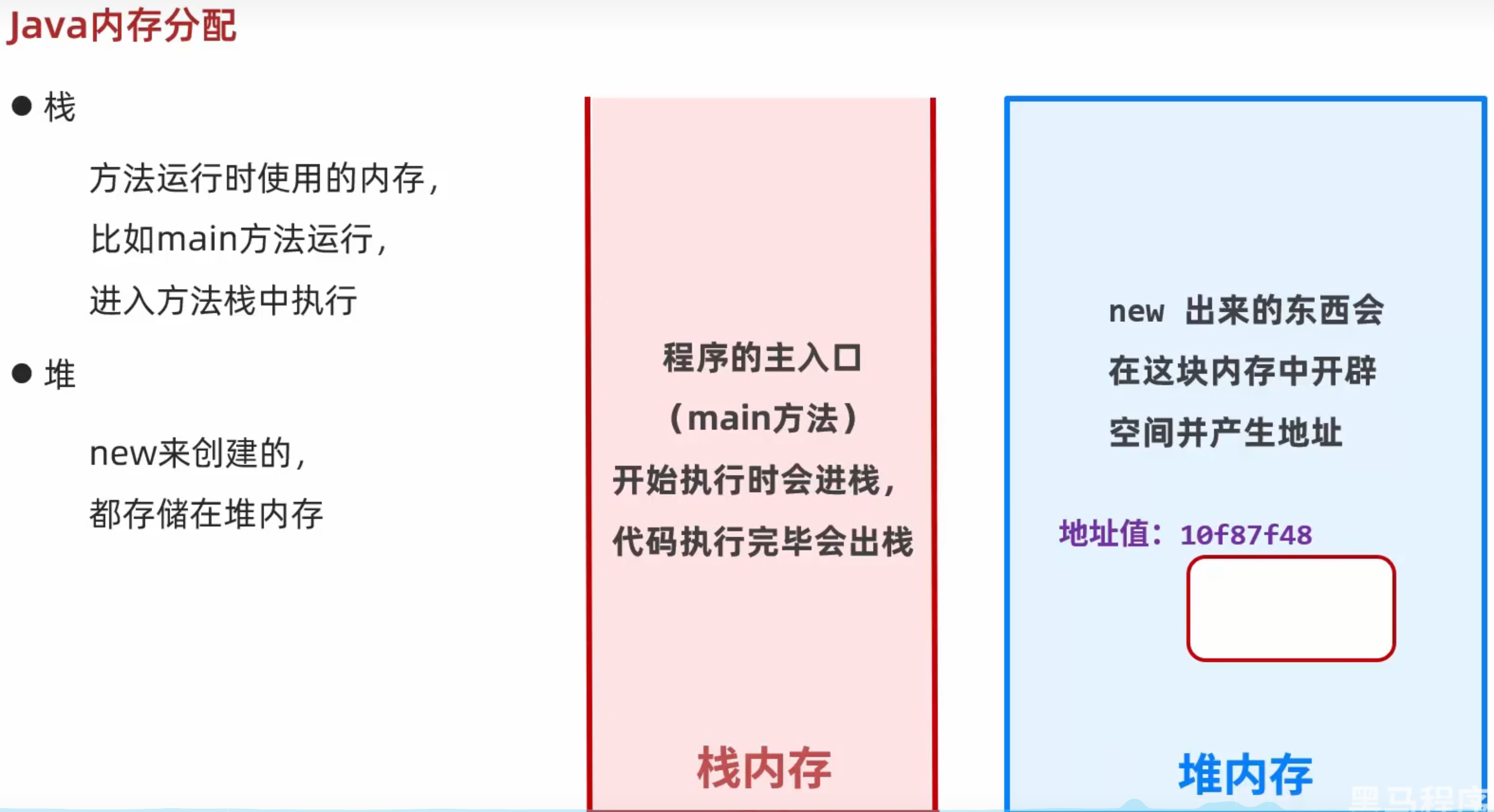
方法
方法的定义格式
1 2 3
| public static void 方法名() { 方法体 }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| 方法名(); public class bianliang1 { public static void main(String[] args) { playGame(); }
public static void playGame() { System.out.println("aa"); System.out.println("BB"); System.out.println("CC"); } } public class bianliang1 { public static void main(String[] args) { plusWhat(); }
public static void plusWhat() { int a = 1; int b = 2; System.out.println(a + b); } }
|
1
| public static void 方法名 (参数1, 参数2....){ }
|
比如:
1 2 3 4 5 6 7 8 9 10 11 12
| public class bianliang1 { public static void main(String[] args) {
plusWhat(1,2); }
public static void plusWhat(int num1, int num2) { int sum = num1 + num2; System.out.println(sum); } }
|
形参和实参
- 形参:全称形式参数,是指方法定义中的参数。
- 实参:全称实际参数,方法调用中的参数。
方法调用时形参实参一一对应。
带返回值方法的定义和调用
1 2 3 4 5 6 7 8
| public static 返回值类型 方法名 (参数){ 方法体 return 返回值; } public static int getSum (int a, int b){ int c = a + b; return c; }
|
1.直接调用
2.赋值调用
3.输出调用
1 2 3 4 5
| 1.我要干嘛? 2.需要什么? 3.方法的调用出,是否需要继续使用方法的结果。 如果需要,则必须有返回值 如果不需要,写不写返回值都行
|
练习:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| public class bianliang1 { public static void main(String[] args) {
int mianji1 = plusWhat(1,2); int mianji2 = plusWhat(3,4); compare(mianji1,mianji2); }
public static int plusWhat(int length, int width) {
int mianji = length * width;
return mianji;
}
public static void compare(int input1, int input2) {
if ( input1 > input2){ System.out.println("第一个更大"); }else { System.out.println("第二个更大"); } return; } }
|
注意事项:
- 方法不调用就不执行
- 方法与方法之间是平级关系,不能互相嵌套定义。
- 方法的编写顺序和执行顺序无关。
- 方法的返回值类型为void,表示该方法没有返回值,没有返回值的方法可以省略return不写。如果非要写return,后面不能跟具体的数据。
- return语句下面不能编写代码,因为永远执行不到。
方法的重载
- 在同一个类中,定义了多个同名的方法,这些同名的方法具有相同的功能。
- 每个方法具有不同的参数类型或参数个数,这些同名的方法就构成了重载关系。
Java虚拟机会通过不同的参数来区分同名的方法,所以重载的关键是要把同种方法不同的形参区分开。
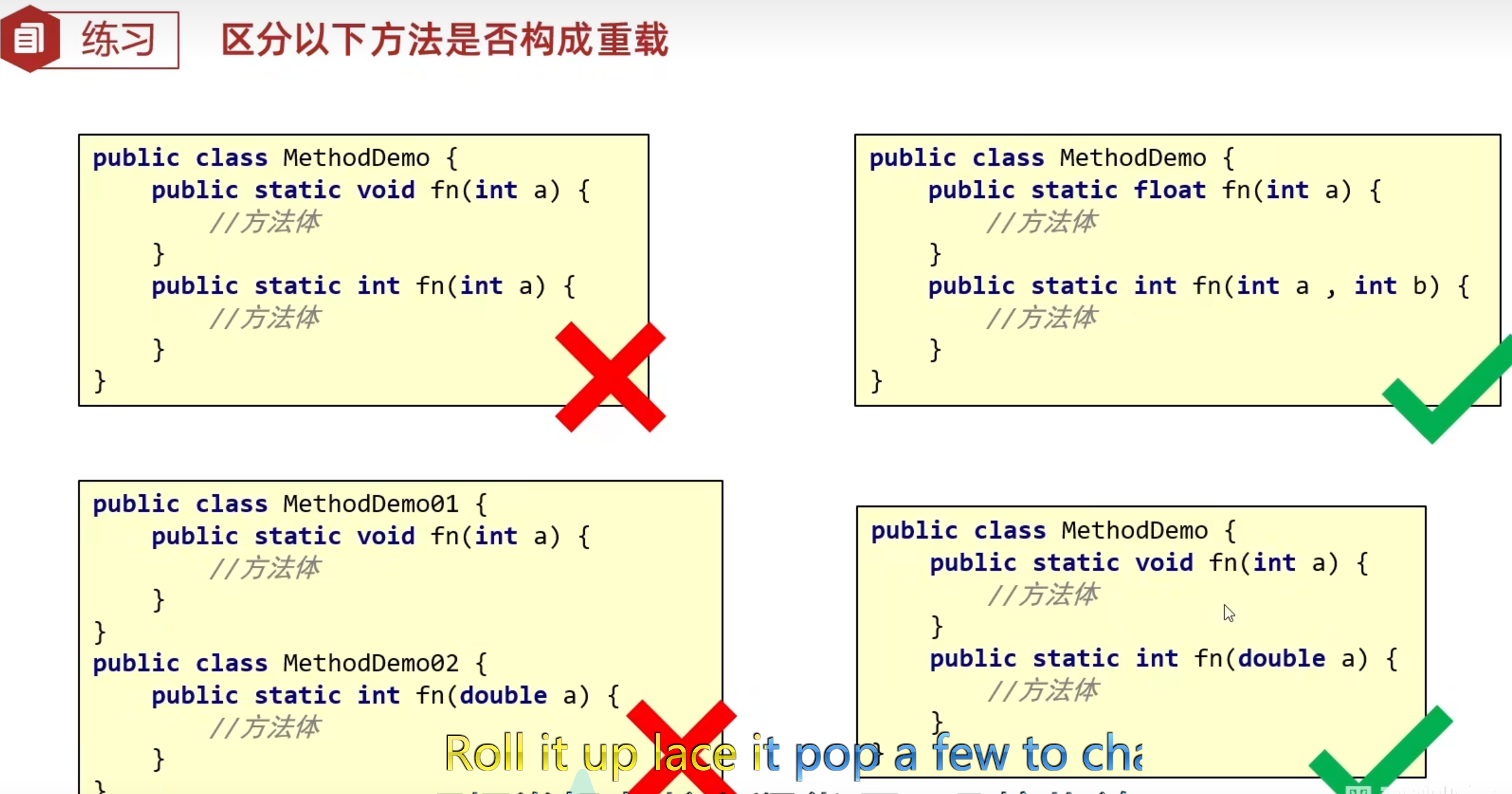
练习:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| public class bianliang1 { public static void main(String[] args) {
}
public static void equalWhat(int num1, int num2) {
if (num1 == num2){ System.out.println("两数相等"); }else { System.out.println("不相同"); }
return; } public static void equalWhat(double num1, double num2) {
if (num1 == num2){ System.out.println("两数相等"); }else { System.out.println("不相同"); }
return; } public static void equalWhat(short num1, short num2) {
if (num1 == num2){ System.out.println("两数相等"); }else { System.out.println("不相同"); }
return; } }
|
练习:遍历一个数组的方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| public class bianliang1 { public static void main(String[] args) {
int[] arr = {1, 2, 3, 4, 5};
equalWhat(arr);
}
public static void equalWhat(int[] arr) {
System.out.print("["); for (int i = 0; i < arr.length; i++) { if (i == arr.length - 1){ System.out.print(arr[i]); }else { System.out.print(arr[i] + ", "); } } System.out.print("]"); return; }
}
|
练习:设计一个方法求数组的最大值,并将最大值返回
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| public class bianliang1 { public static void main(String[] args) {
int[] arr = {1, 2, 3, 4, 5};
equalWhat(arr);
}
public static void equalWhat(int[] arr) { int max = arr[0];
for (int i = 0; i < arr.length; i++) {
if (arr[i] > max){ max = arr[i]; } } System.out.print("该数组的最大值是:" + max); return; }
}
|
练习:定义一个方法判断数组中的某一个数是否存在,将结果返回
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| public class bianliang1 { public static void main(String[] args) {
int[] arr = {1, 2, 3, 4, 5};
int target = 5;
boolean flag = equalWhat(arr, target);
System.out.println(flag); }
public static boolean equalWhat(int[] arr, int target) {
for (int i = 0; i < arr.length; i++) {
if (arr[i] == target){
return true; } } return false; }
}
|
练习:复制数组
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| public class bianliang1 { public static void main(String[] args) {
int[] arr = {1, 2, 3, 4, 5,6,7,8,9};
int from1 = 1;
int to1 = 8;
int[] flag = copyWhat(arr, from1, to1);
for (int i = 0; i < flag.length; i++) {
System.out.println(flag[i]);
}
}
public static int[] copyWhat(int[] arr1, int from, int to) {
int[] arr2 = new int[to - from];
for (int i = from, j = 0; i < to && j < arr2.length; i++ , j++) {
arr2[j] = arr1[i]; } return arr2; }
}
|
方法的内存
整数类型,浮点数类型,布尔类型,字符类型(数据值存储在自己的空间中)
除了基本数据类型的其它所有类型(变量中存储的是地址值)。
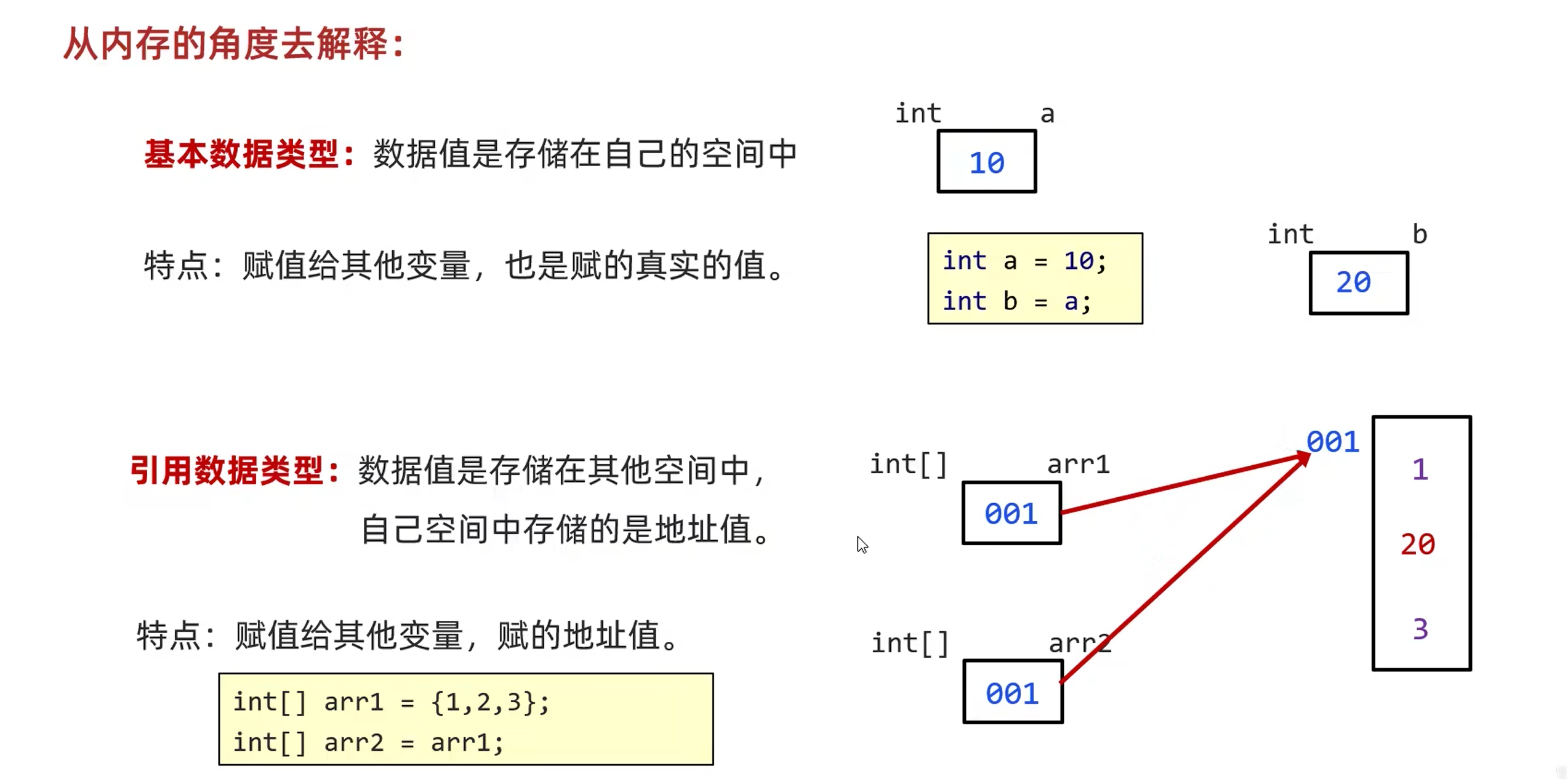
方法传递基本数据类型的内存原理
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| public class bianliang1 { public static void main(String[] args) { int num1 = 100;
System.out.println("调用change方法前值为:" + num1);
change(num1);
System.out.println("调用change方法后值为:" + num1);
}
public static void change(int numwhat) {
numwhat = 200;
return; }
}
100 100
|
原因参考:
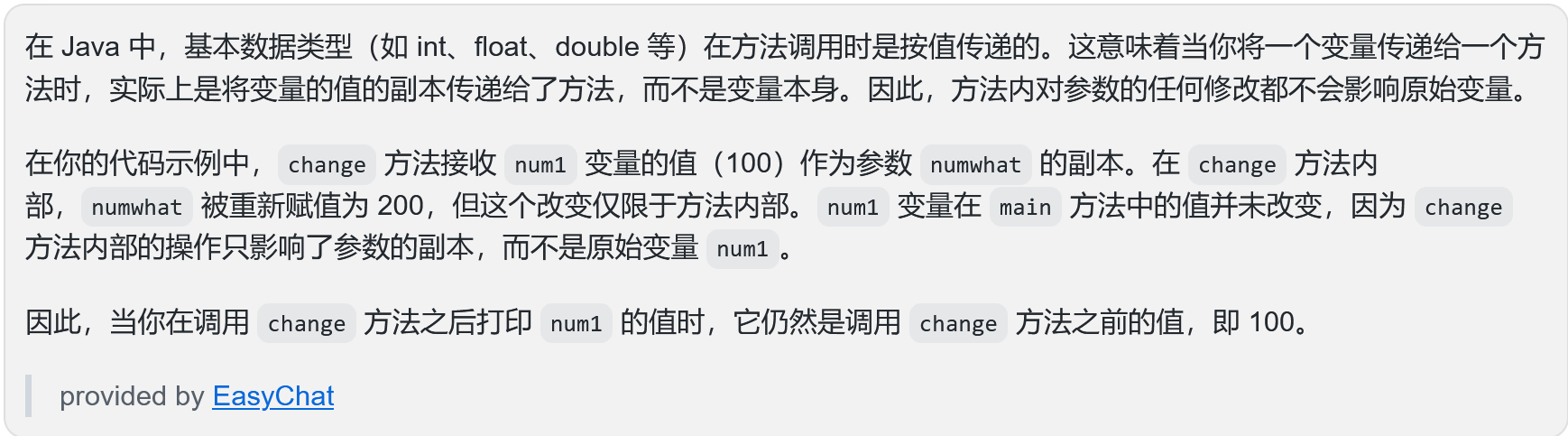
当然,如果想通过调用change
方法将num
的值改变,可以:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| public class bianliang1 { public static void main(String[] args) { int num1 = 100;
System.out.println("调用change方法前值为:" + num1);
num1 = change(num1);
System.out.println("调用change方法后值为:" + num1);
}
public static int change(int numwhat) {
numwhat = 200;
return numwhat; }
}
100 200
|
如果对于引用数据类型:传递引用数据类型时。传递的是地址值,形参的改变会影响实际参数的值。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| public class bianliang1 { public static void main(String[] args) { int[] arr1 = {10, 20, 30};
System.out.println("调用change方法前值为:" + arr1[1]);
change(arr1);
System.out.println("调用change方法后值为:" + arr1[1]);
}
public static void change(int[] numwhat) {
numwhat[1] = 200;
return; }
}
20 200
|
综合练习部分
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
| import java.util.Scanner;
public class bianliang1 { public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入当前月份:");
int month = sc.nextInt();
System.out.println("请输入目标机票:");
String type = sc.next();
int expensive = 1000;
int cheap = 500;
int money = 0;
if (type == "头等舱") {
money = expensive;
} else { money = cheap; }
if (5 <= month && month <= 10) {
System.out.println("芜湖!目前是淡季,机票价格五折!");
double cost = money * 0.5;
} else { System.out.println("芜湖!目前是旺季,机票不打折");
double cost = money; }
System.out.println("请支付" + money + "元");
}
}
|
唉,看了标准答案,感觉我像脑残
P74 - P81
面向对象
面向对象介绍
获取/设计已有对象并使用
设计对象并使用
类:对象共同特征的描述
对象:真实存在的具体东西
java中,必须先设计类,才能获得对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| public class 类名{ 1.成员变量(代表属性,一般是名词) 2.成员方法(代表行文,一般是动词) 3.构造器 4.代码块 5.内部类 }
public class Phone { String Name;
double Price; public void call(){} public void playGame(){} }
|
类名 对象名 = new 类名();
Phone p = new Phone();
访问属性:对象名.成员变量
访问行为:对象名.方法名
用来描述一类事物的类,专业叫做:javabean
类。在该类中,不写main
方法。
编写main
方法的类叫测试类。
一个java文件中可以定义多个class
类,但只能有一个类是public
修饰。而且public
修饰的类名必须是代码文件名。不过实际开发中建议一个文件定义一个class
类。
成员变量的完整定义格式是:修饰符 数据类型 变量名称 = 初始化值; 一般无需指定初始化值,存在默认值。
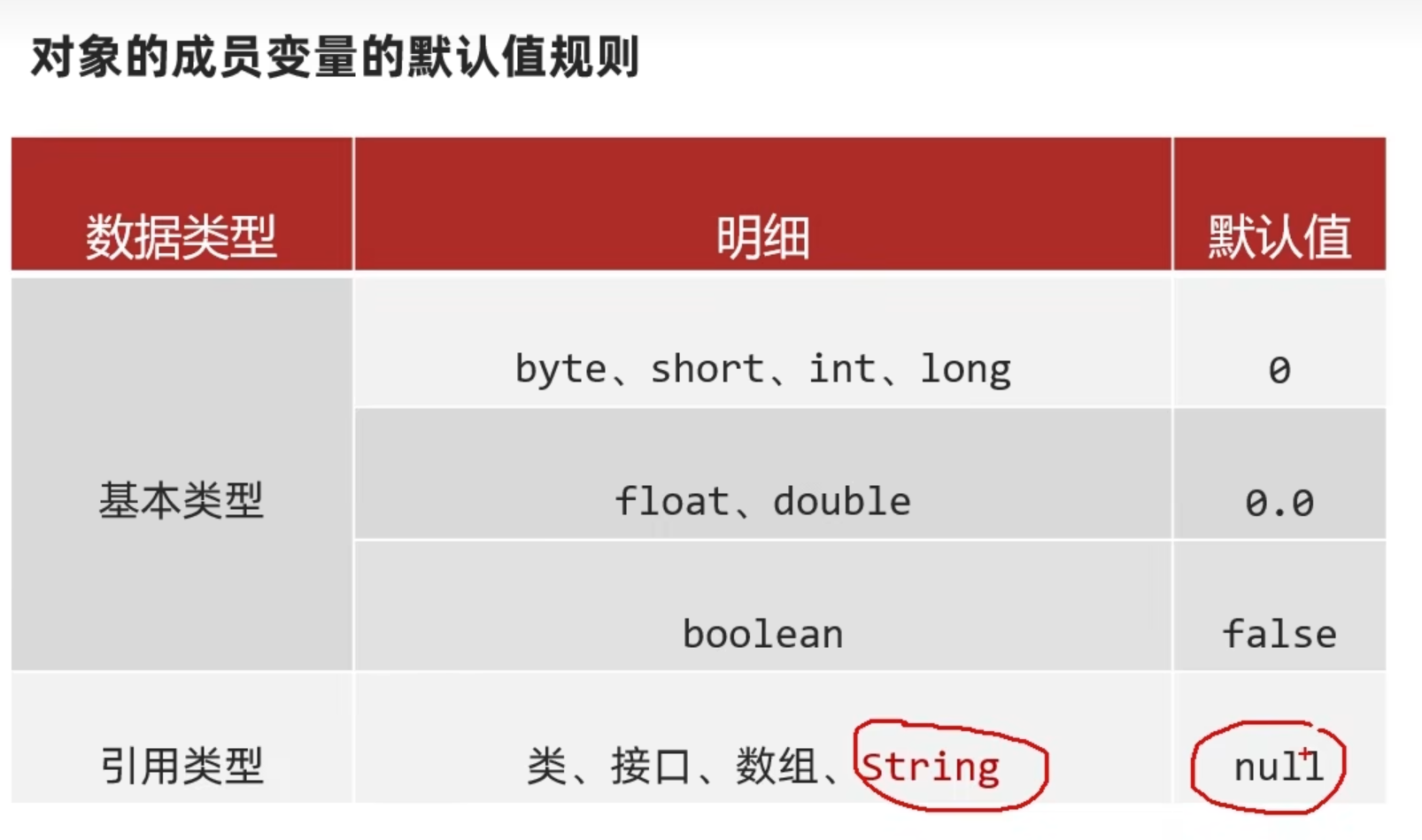
练习:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| public class girlFriend { String name;
int age;
public void sleep(){ System.out.println("sleeping"); } public void eat(){ System.out.println("eating"); } }
public class testgril { public static void main(String[] args) { girlFriend hername = new girlFriend();
hername.age = 21; hername.name = "what?"; hername.eat(); hername.sleep(); System.out.println(hername.age); System.out.println(hername.name);
} }
|
封装
面向对象三大特征:封装,继承,多态。
封装:对象代表什么,就得封装对应的数据,并提供数据对应的行为。
告诉我们,如何正确设计对象的属性和方法。
已经设计好的类,其中类的内部细节被隐藏,而通过公共接口提供对这些细节的访问和修改。这有助于实现类的安全性和灵活性。
private关键字
- 是一个权限修饰符
- 可以修饰成员(成员变量和成员方法)
- 被private修饰的成员只能在本类中才能访问
1 2 3 4 5 6 7 8 9
| public class GirlFriend { private String name; private int age; private String gender; } GirlFriend gf1 = new GirlFriend(); gf1.age = 18;
|
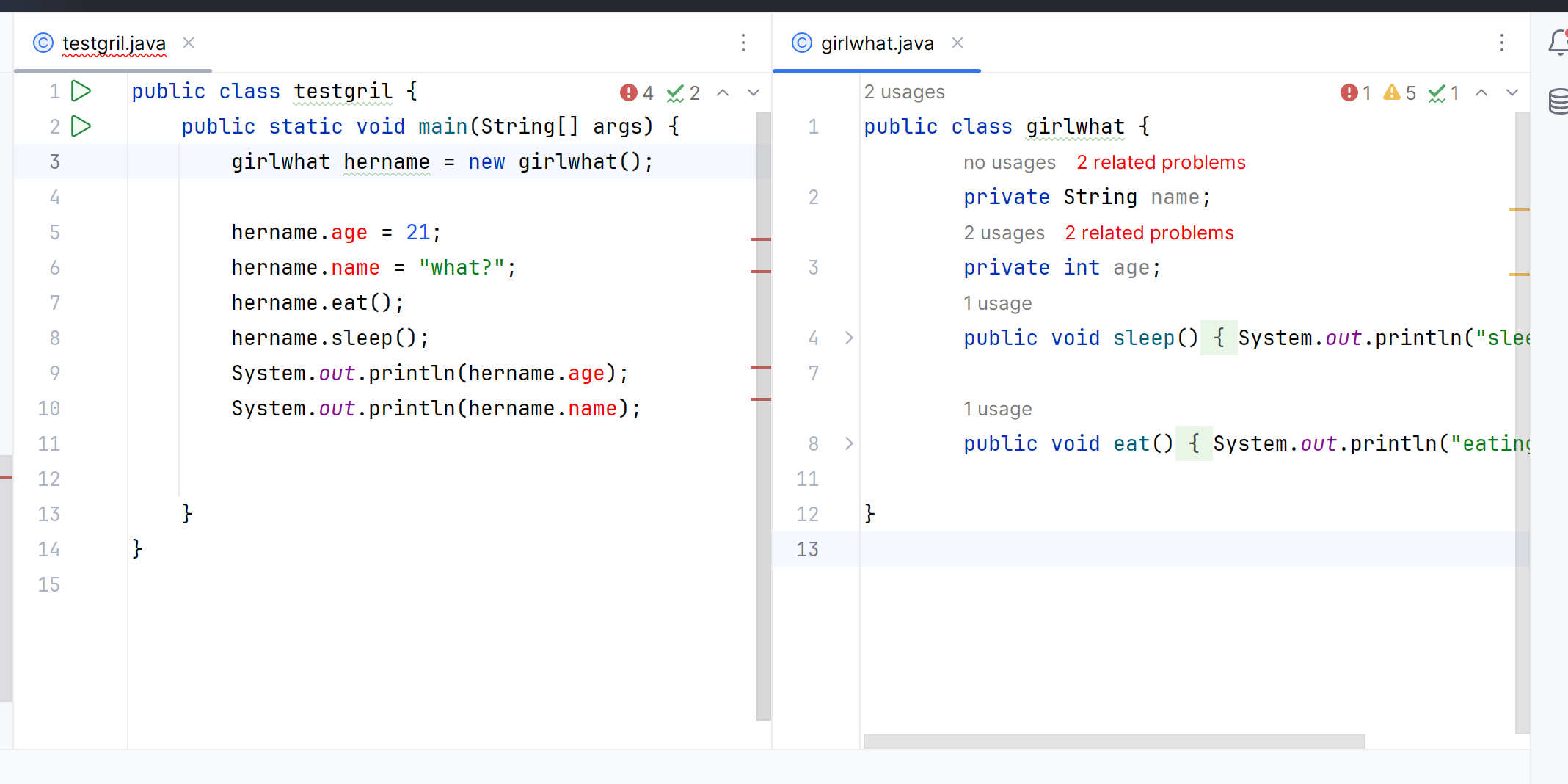
可以这样修改:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| public class girlwhat { private String name; private int age;
public void setName(String n){ name = n; } public String getName(){ return name; } public void setAge(int a){ if (a > 18 && a < 30){ age = a; }else { System.out.println("非法参数"); } return; } public int getAge(){ return age; } public void sleep() { System.out.println("sleeping"); }
public void eat() { System.out.println("eating"); }
}
public class testgril { public static void main(String[] args) { girlwhat hername = new girlwhat();
hername.setName("txy"); System.out.println(hername.getName()); hername.setAge(18); System.out.println(hername.getAge()); hername.eat(); hername.sleep();
} }
|
this关键字
对于如下代码:
1 2 3 4 5 6 7 8
| public class testtest { public int age; public void method(){ int age = 10; System.out.println(age); } }
|
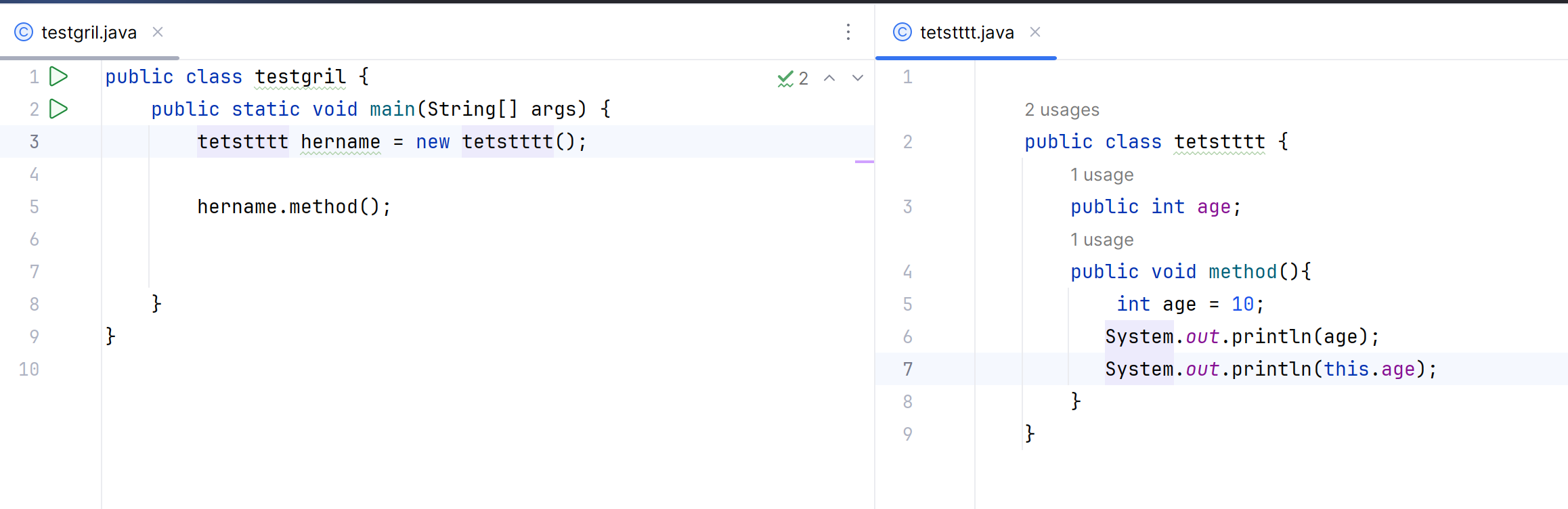
this
关键字可以定位到成员变量,也可以通过就近原则:
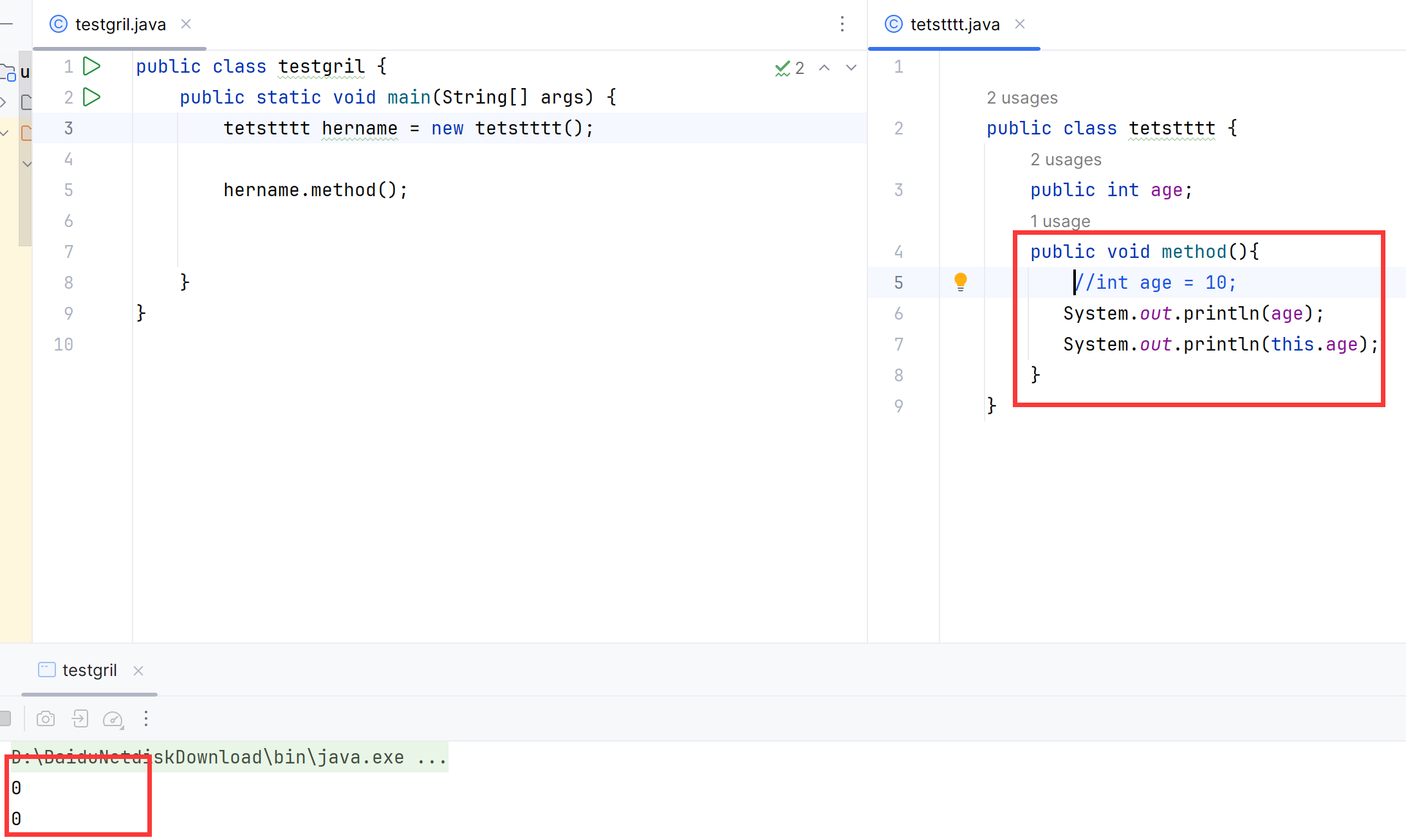
此时输出的都是成员变量。
所以上面的代码可以进行如下更改:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| public class girlwhat { private String name; private int age;
public void setName(String name){ this.name = name; } public String getName(){ return name; } public void setAge(int age){ if (age > 18 && age < 30){ this.age = age; }else { System.out.println("非法参数"); } return; } public int getAge(){ return age; }
|
注意两个get
方法,加不加this
都行。
总结:this
关键字就是指成员变量(避免和局部变量混淆)。当然也可以通过就近原则避免这个问题。
构造方法
- 概述:也叫做构造器、构造函数。
- 作用:在创建对象时给成员变量进行初始化。
比如对于:
1 2 3 4 5
| public class StudentDemo { public static void main(String[] args){ Student s1 = new Student(); } }
|
构造方法的格式:
1 2 3 4 5 6 7
| public class Student {
修饰符 类名(参数) { 方法体; } }
|
懒得打字了。。直接贴图片了
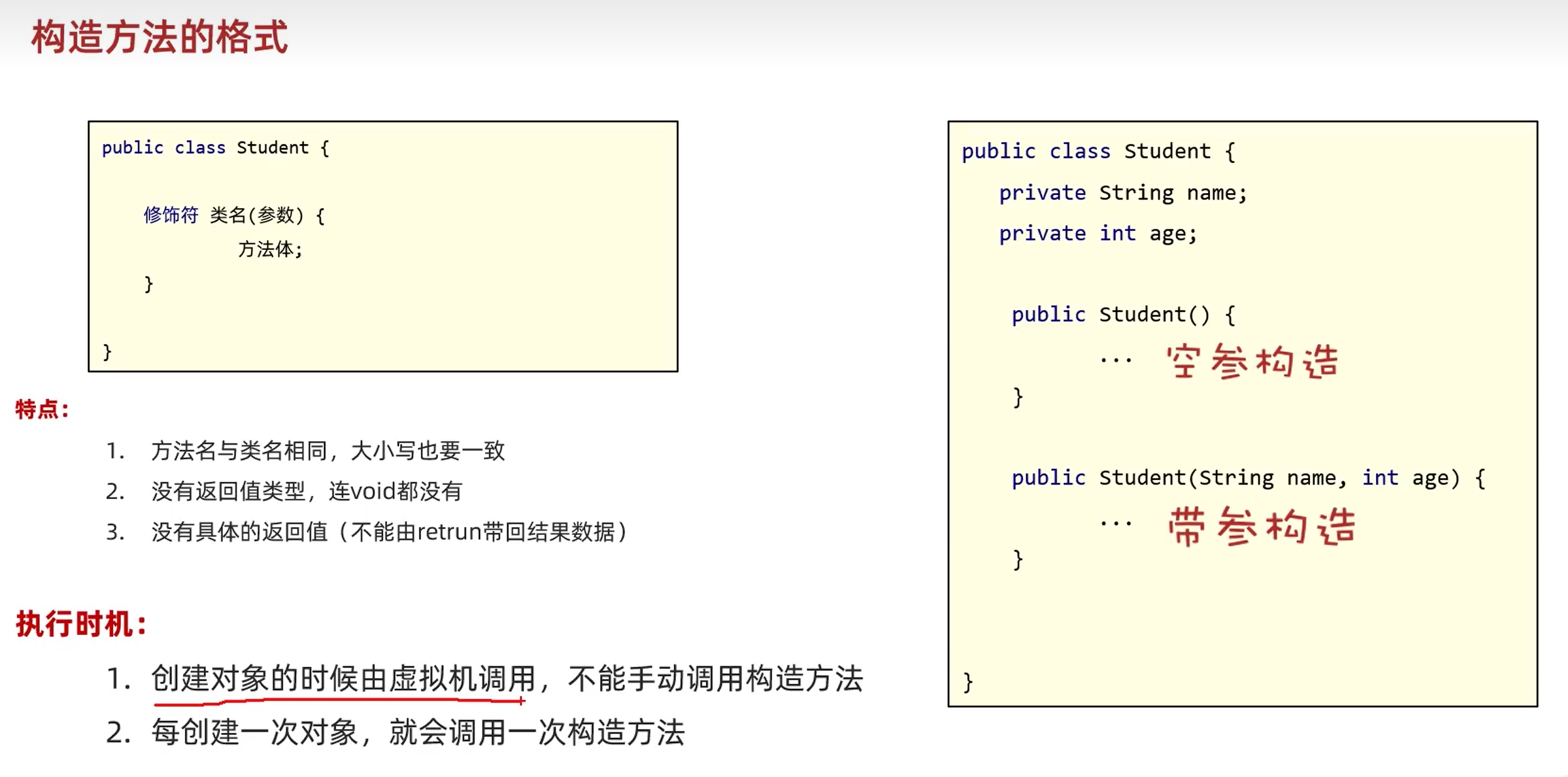
空参构造:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| public class testgril { public static void main(String[] args) { girlwhat hername = new girlwhat();
} }
public class girlwhat { private String name; private int age; public girlwhat(){ System.out.println("芜湖!我是空参构造"); } }
|
当然,我们也可以利用有参构造在创建对象的时候就给成员变量赋值:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| public class girlwhat { private String name; private int age; public girlwhat(){ System.out.println("芜湖!我是空参构造"); } public girlwhat(String name, int age){ this.name = name; this.age = age; System.out.println("你使用了有参构造"); }
public class testgril { public static void main(String[] args) { girlwhat hername = new girlwhat(); girlwhat hername2 = new girlwhat("txy",22); System.out.println(hername2.getName()); System.out.println(hername2.getAge()); } }
芜湖!我是空参构造 你使用了有参构造 txy 22
|
构造方法注意事项:
如果没有定义构造方法,系统将会给出一个默认的无参数构造方法。
如果定义了构造方法,系统将不再提供默认的构造方法。(比如你已经定义了一个有参构造,此时再调用无参构造系统就会报错)。
构造方法的重载:带参构造方法和无参构造方法,两者方法名相同但参数不同,这叫构造方法的重载。
推荐使用方式:无论是否使用,都手动书写两种构造方法。
总结:
- 构造方法的作用?初始化成员变量。
- 构造方法有几种?作用?两种,有参构造和无参构造。
- 构造方法有哪些注意事项?写的时候两种构造都要写好。
标准JavaBean
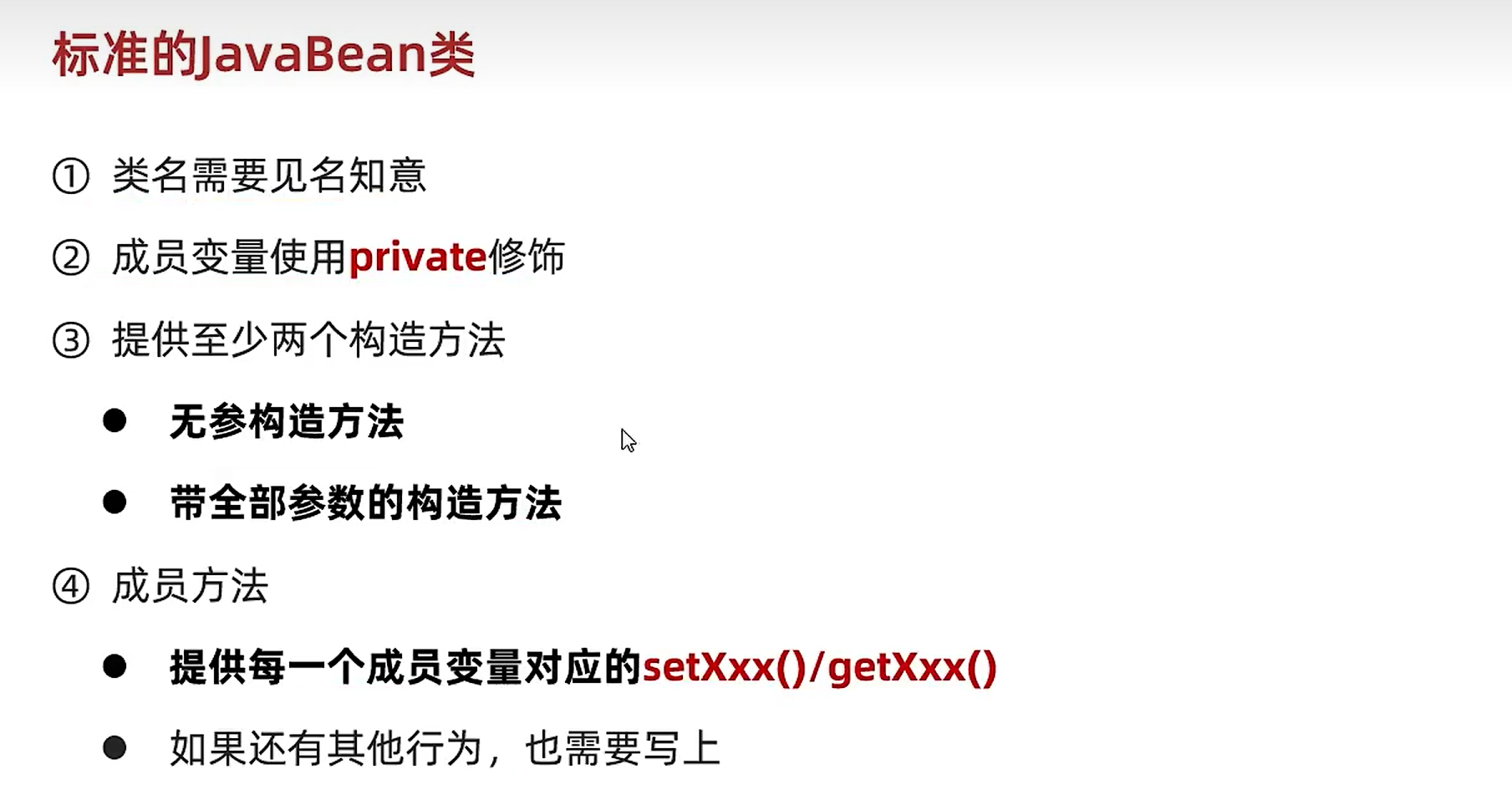
练习:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| public class beanbean { private String name; private String passwd; private String email; private int age; public beanbean(){ System.out.println("我是无参构造!"); } public beanbean(String name, String email, int age, String passwd){ this.name = name; this.email = email; this.age = age; this.passwd = passwd; } public void setName(){ this.name = name; return; } public String getName(){ return name; } public void setEmail(){ this.email = email; return; } public String getEmail(){ return email; } public void setAge(){ this.age = age; return; } public int getAge(){ return age; } public void setPasswd(){ this.passwd = passwd; return; } public String getPasswd(){ return passwd; } }
public class beanwhat { public static void main(String[] args) { beanbean b1 = new beanbean(); beanbean b2 = new beanbean("txy", "q@q", 22, "shit");
System.out.println(b2.getName()); System.out.println(b2.getAge()); System.out.println(b2.getEmail()); System.out.println(b2.getPasswd()); } }
|
快捷生成有参或无参构造:alt + insert
选择construct
。也能通过选getandset
去快速生成方法。
或者通过PTG
插件,写完属性直接右键点击就好。
p87 java内存介绍
基本数据类型和引用数据类型
之前的记忆:
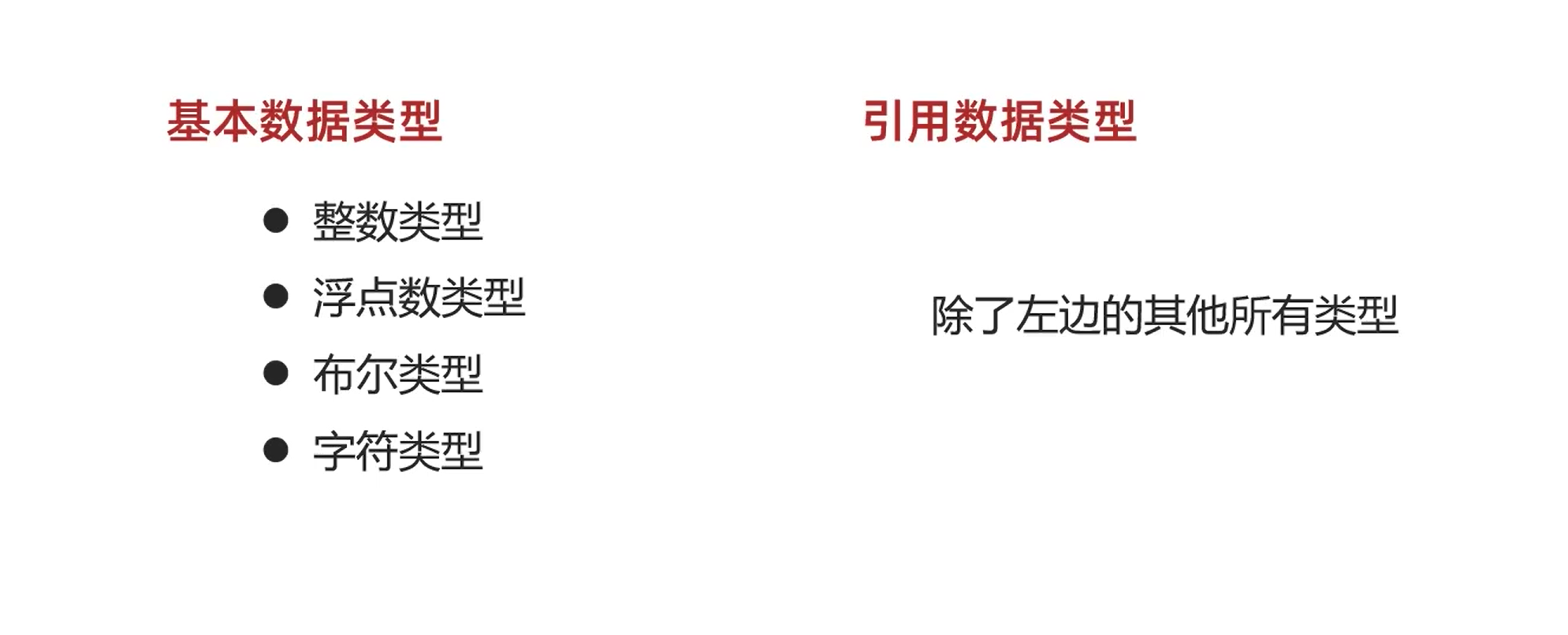
从内存的角度去理解:
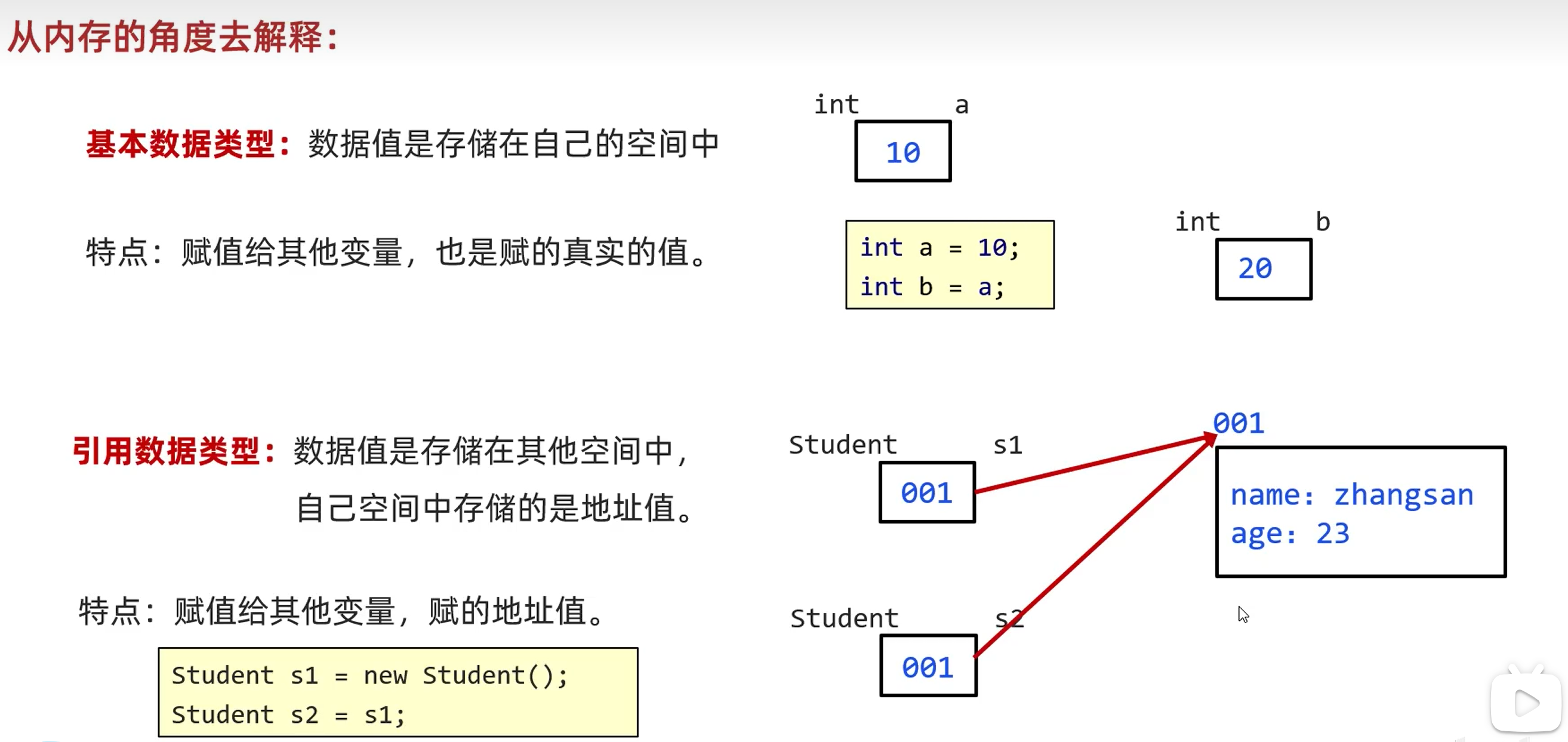
this的内存原理
p89
成员变量和局部变量的区别
- 成员变量:类中方法外的变量
- 局部变量:方法中的变量
面向对象综合练习
P91-P95
P124:
继承
封装
对象代表什么,就得封装相应的数据,并提供数据对应的行为。
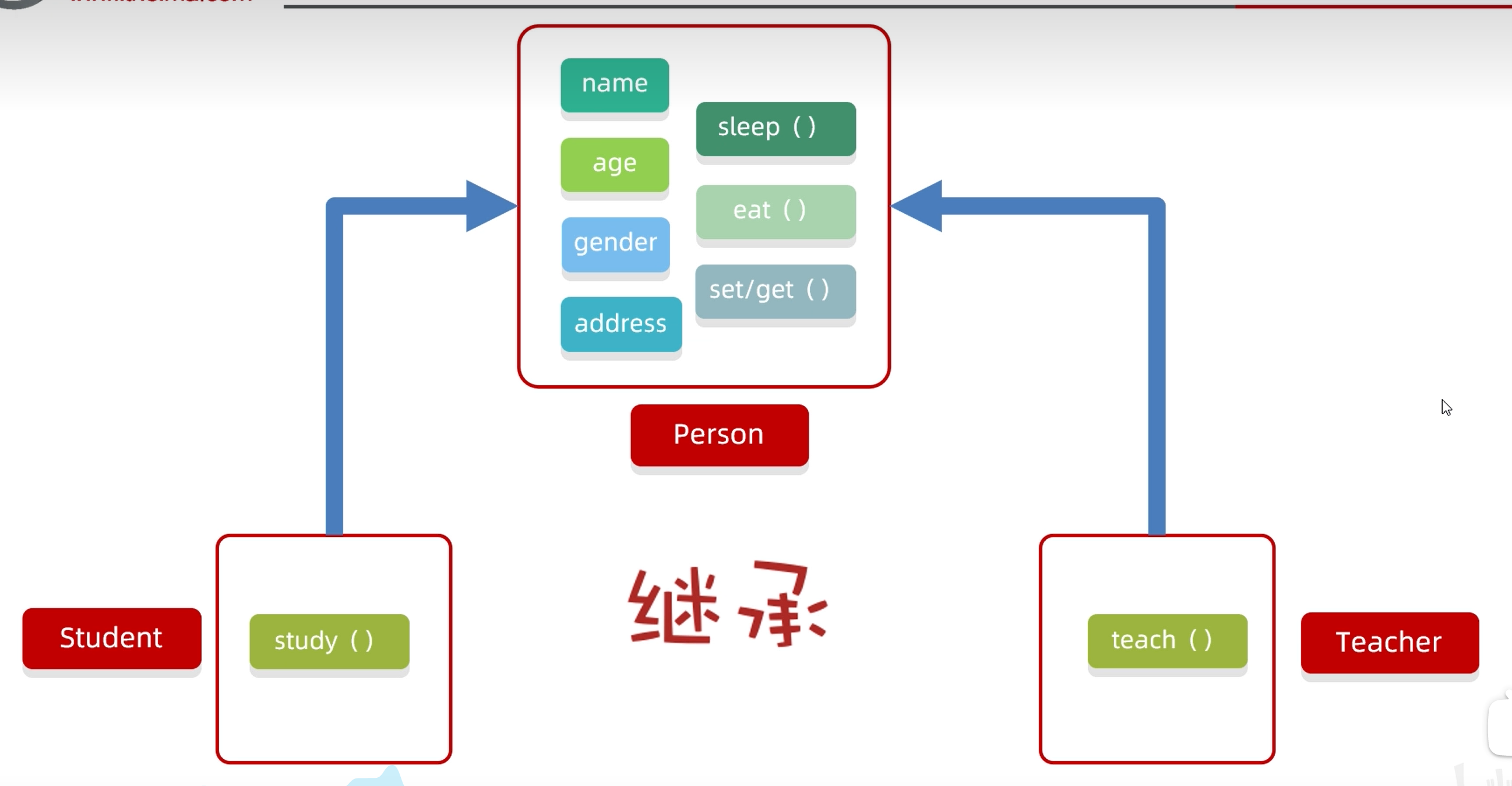
- Java中提供一个关键字extends,用这个关键字可以让一个类和李谷一各类建立起继承关系。
1
| public class Student extends Person{}
|
- Student成为子类(派生类),Person成为父类(基类或者超类)。
使用继承的好处:
- 可以把多个子类中重复的代码抽取到父类中,提高代码的复用性。
- 子类可以在父类的基础上,增加其他的功能,使子类更加强大。
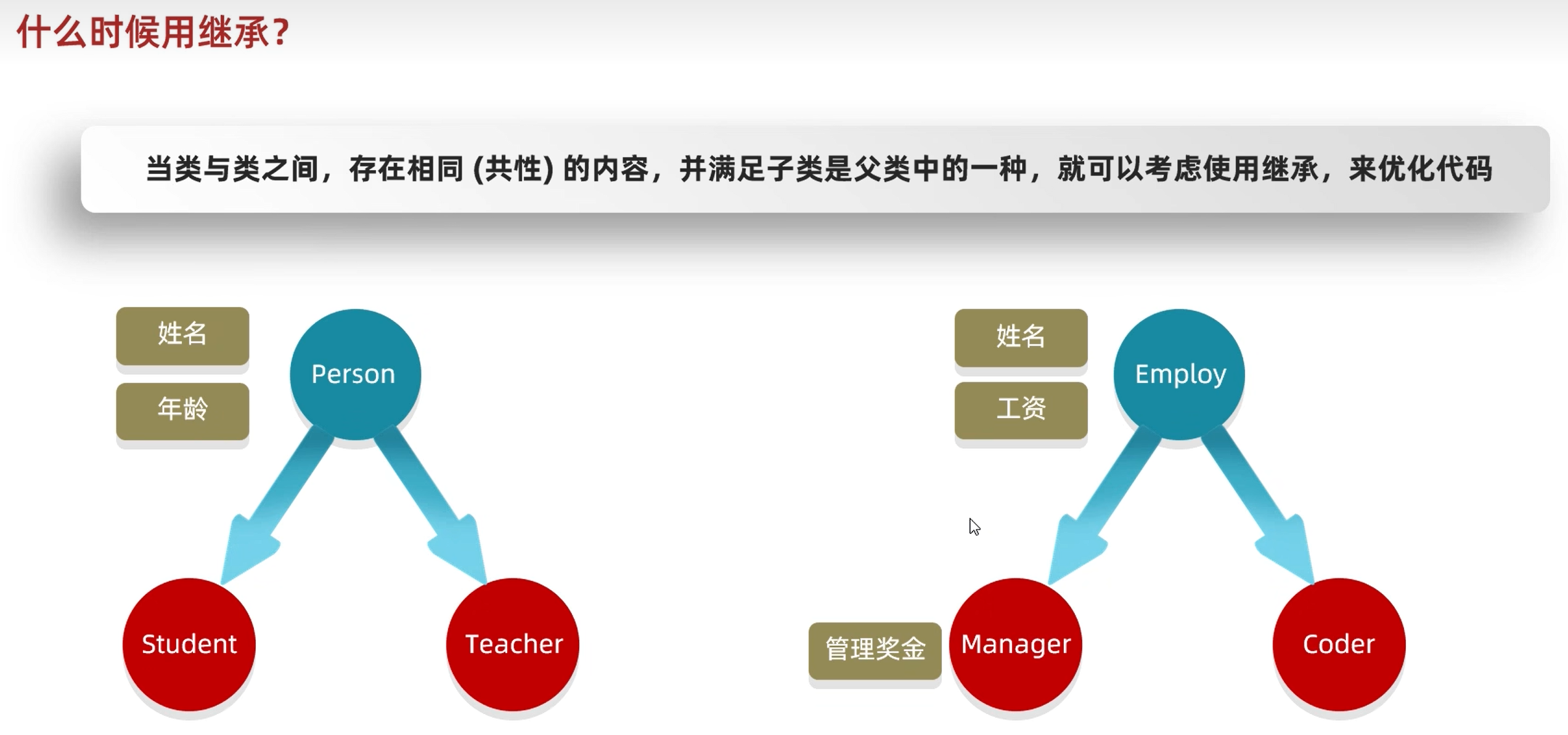
继承的特点:
Java只支持单继承,不支持多继承,但支持多层继承。
单继承:一个子类只能及成果一个父类。
不支持多继承:子类不能同时继承多个父类。
多层继承:子类A继承父类B,父类B可以再继承父类C。
每个类都直接或者间接的继承于Object。
需注意子类只能访问父类中非私有的成员。
P126-128
P129
多态
1 2 3 4
| Student s = new Student();
Person p = new Student();
|
但这东西有什么用?
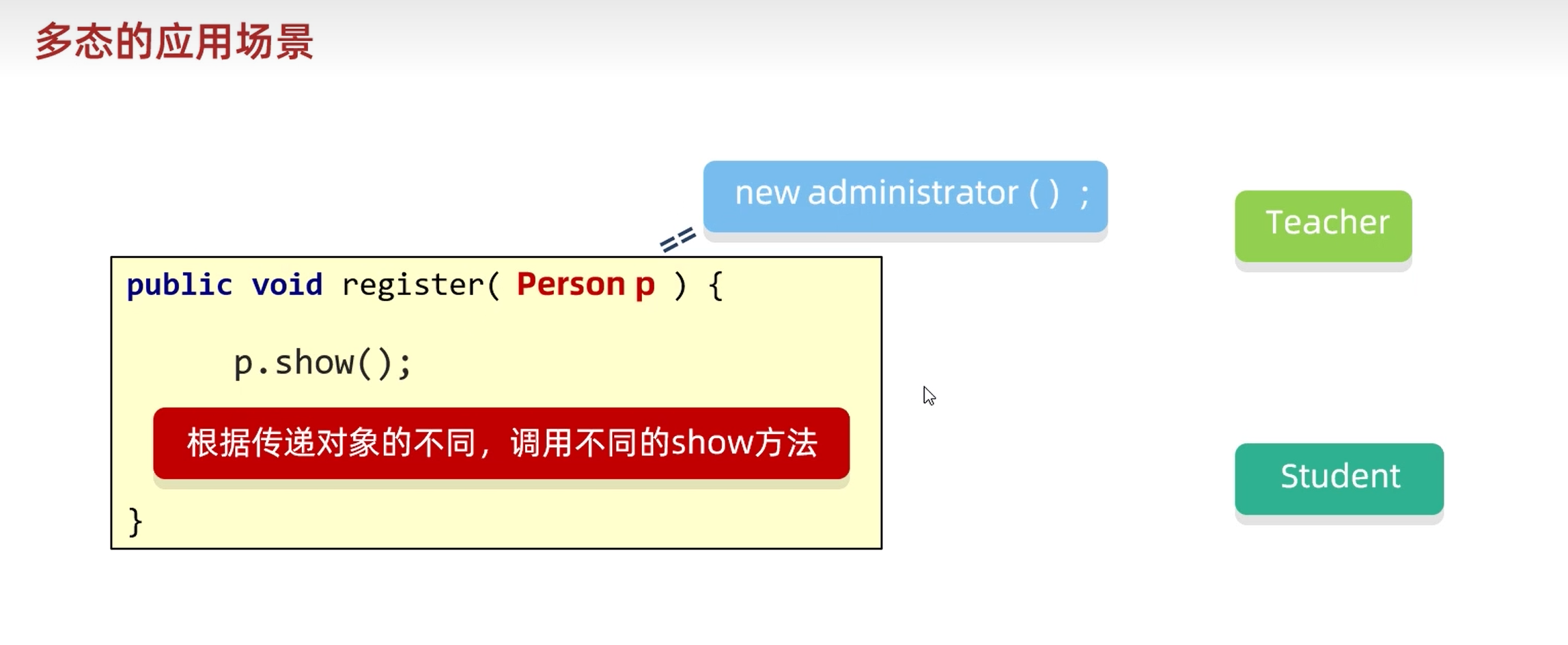
什么是多态?
同类型的对象,表现出的不同形态。
多态的表现形式
父类类型 对象名称 = 子类对象。
多态的前提
有继承/实现关系;
有父类引用指向子类对象( Fu f = new Zi();
)
有方法重写。
如果某方法接收的参数是一个父类,那么可以给他传所有父类下的子类对象。
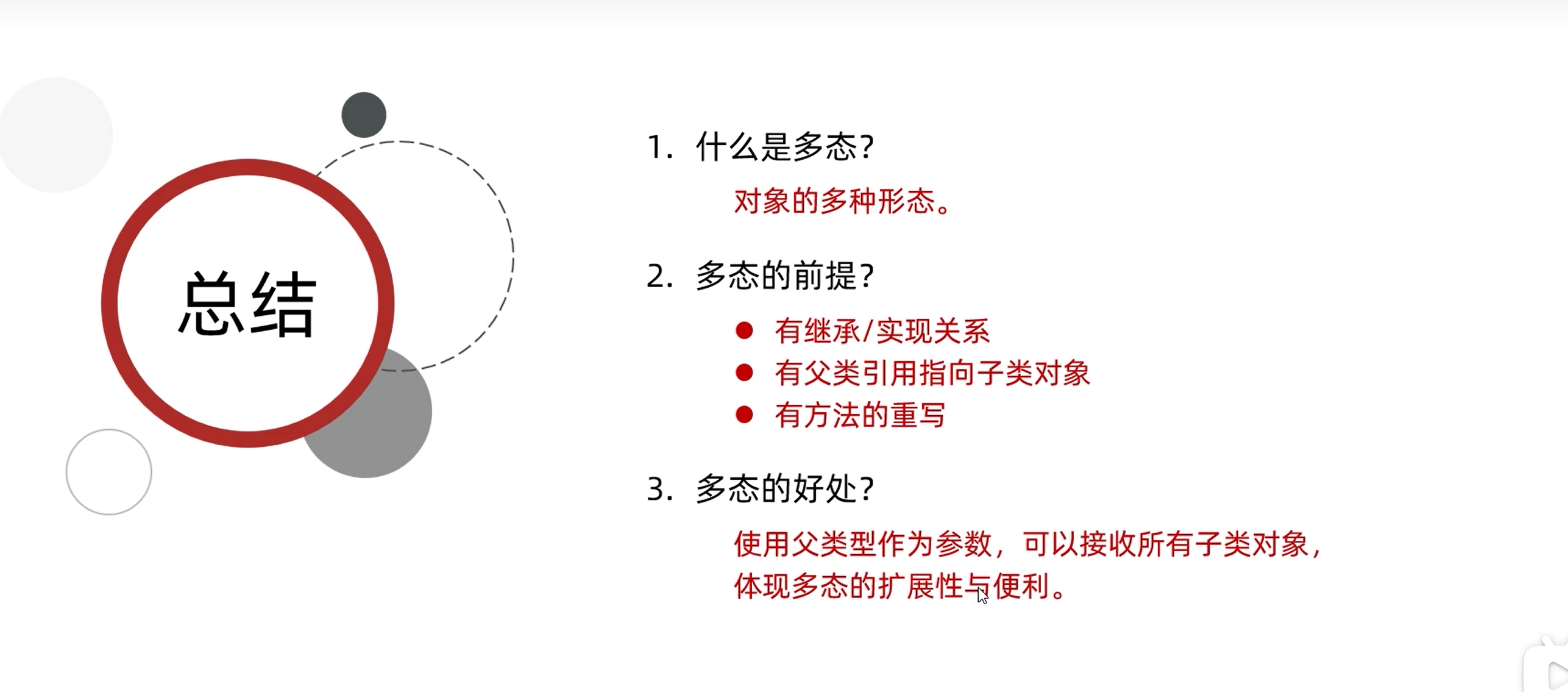
P131=132
包和final
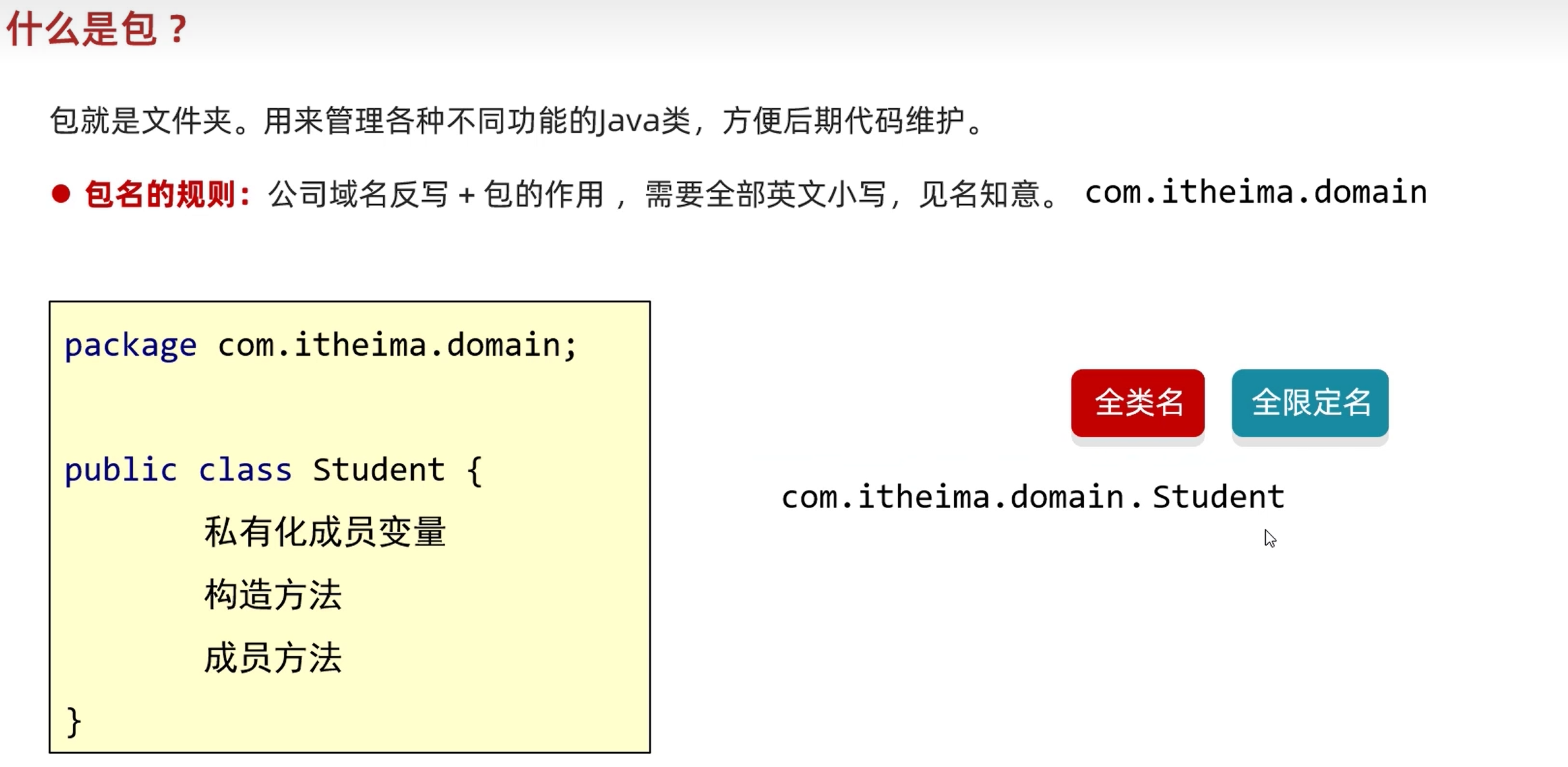
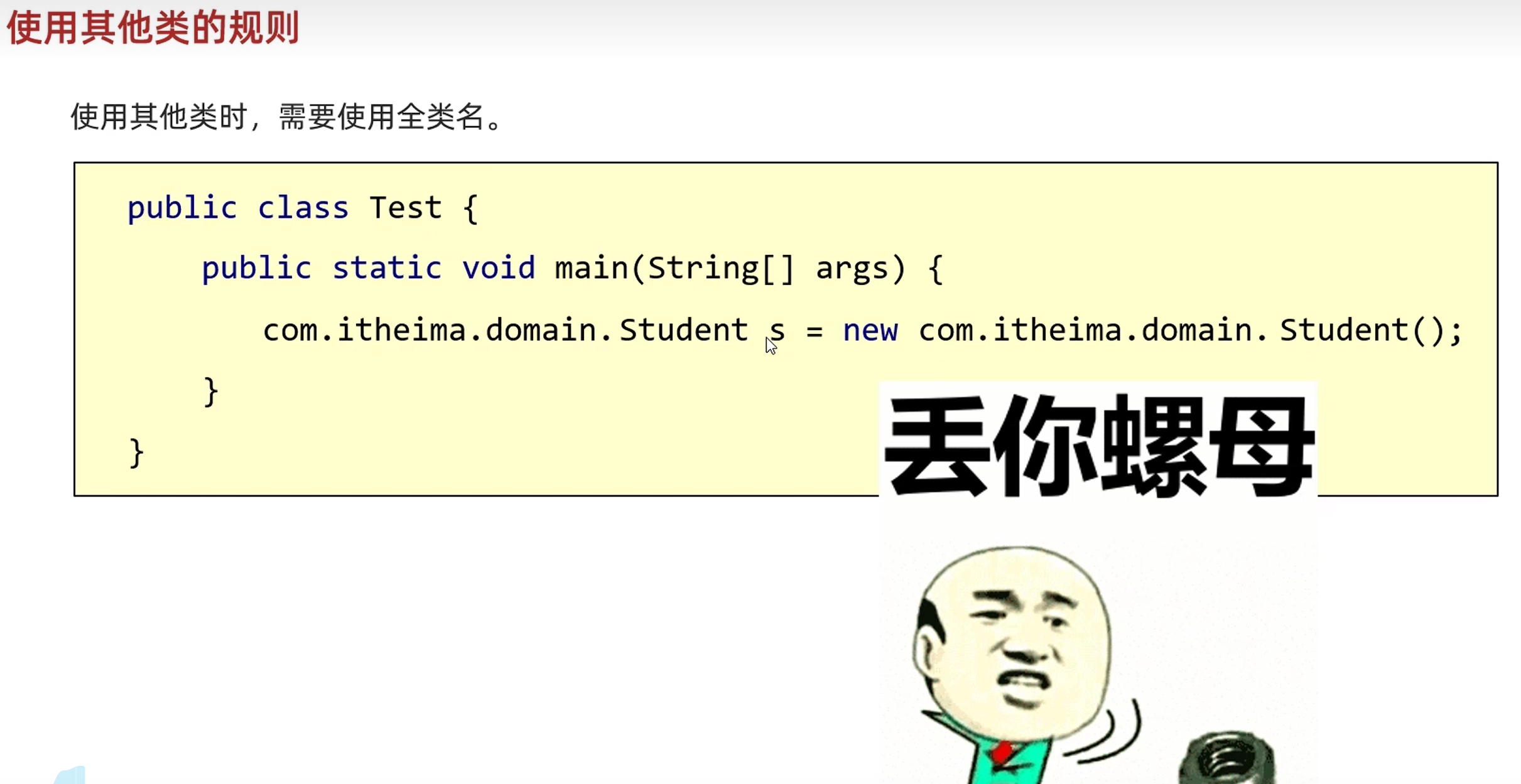
导包:
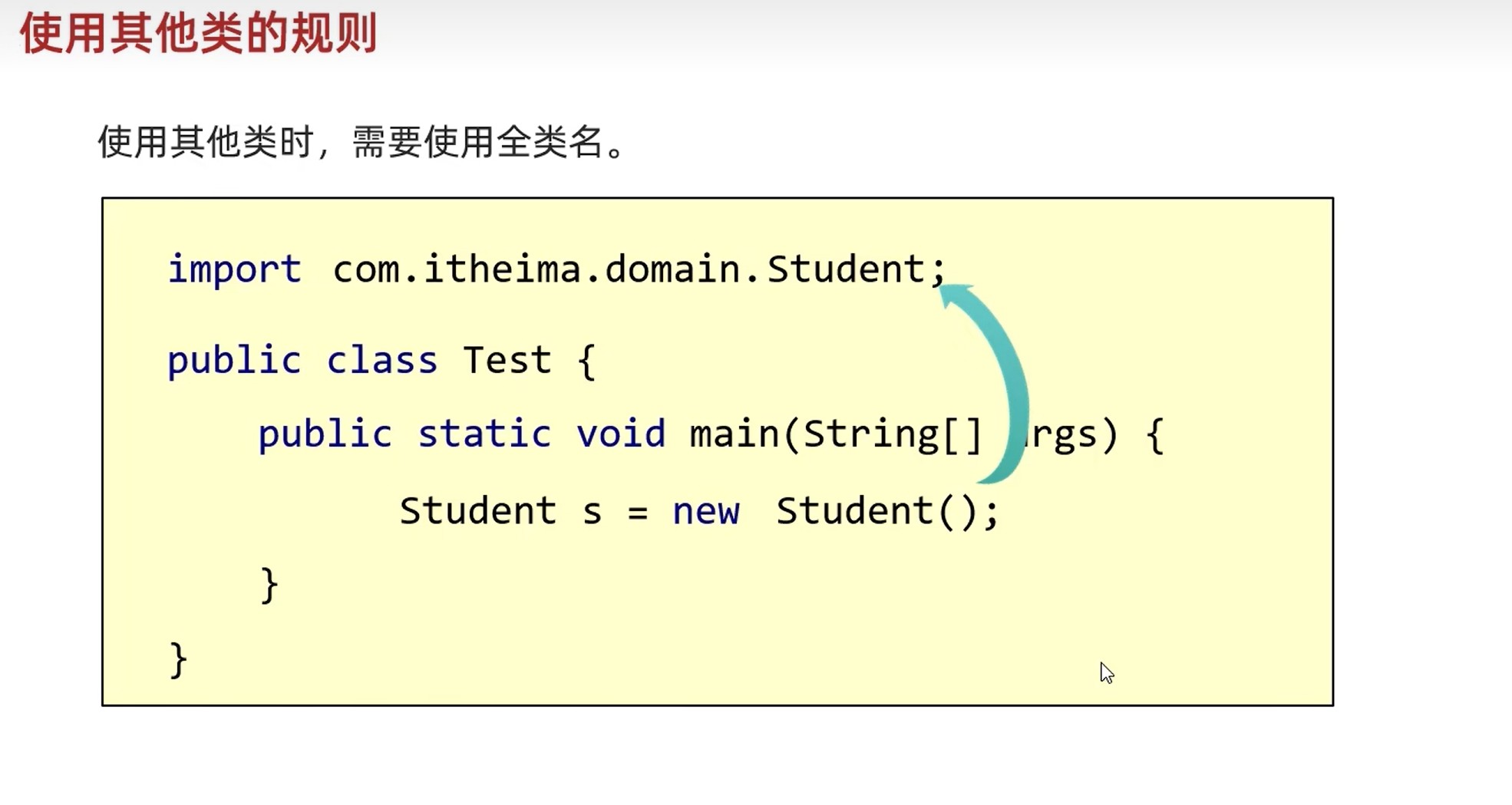
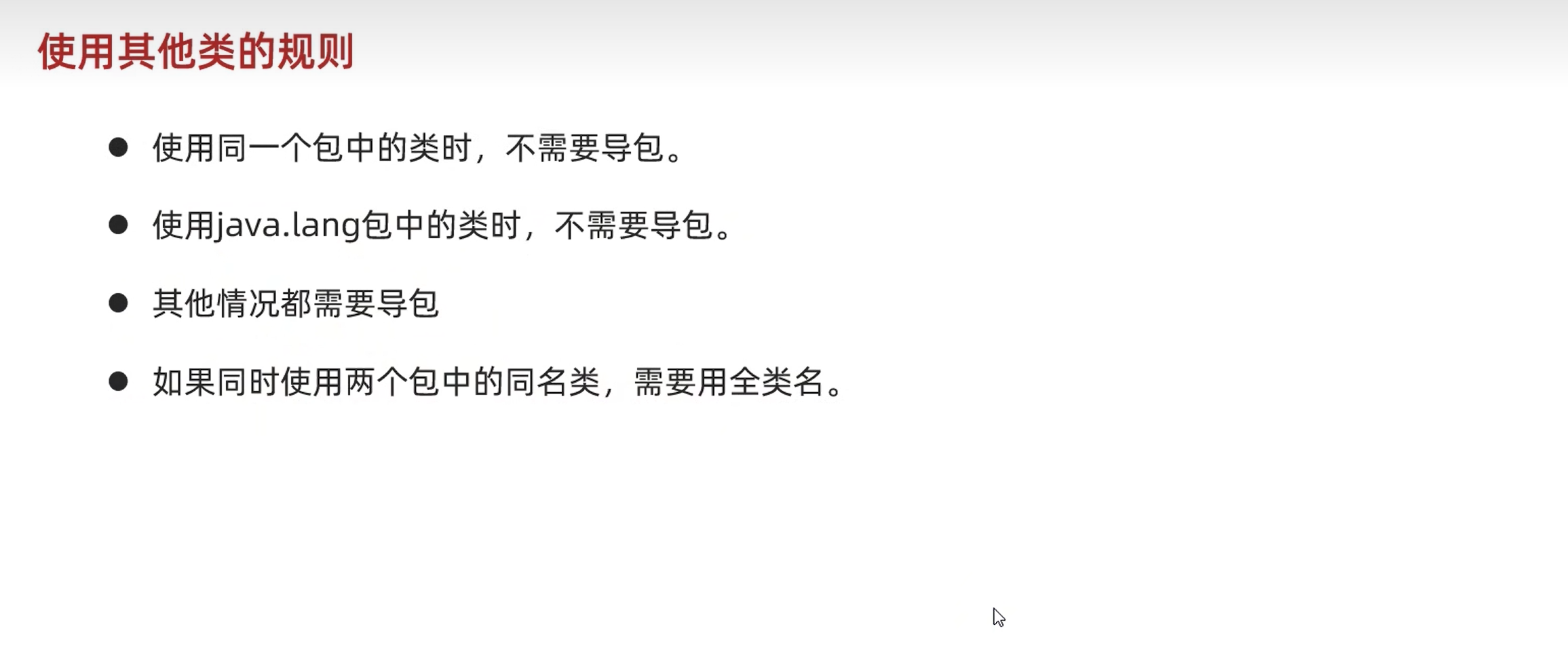
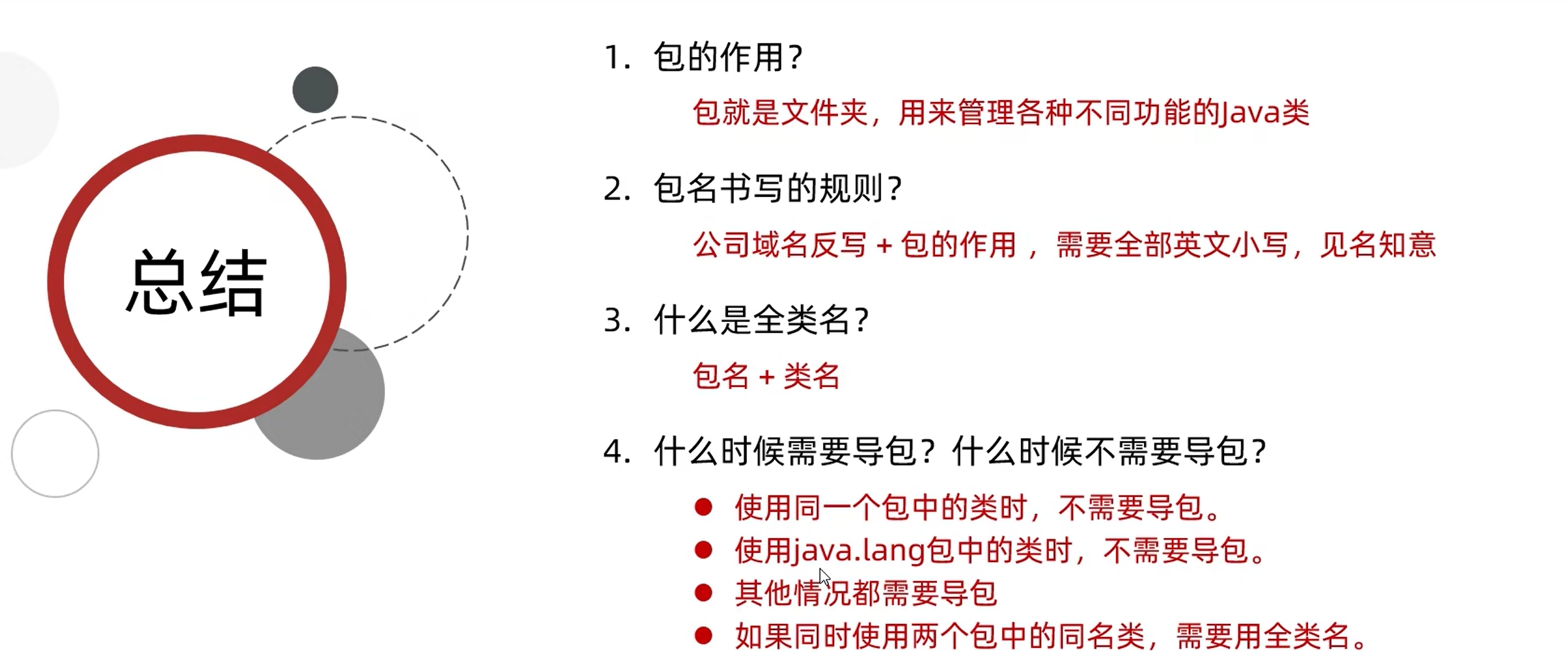
final
- 修饰方法:表明该方法是最终方法,不能被重写。
- 类:表明该类是最终类,不能被继承。
- 变量:叫做常量,只能被赋值一次。
1 2 3 4 5 6 7 8 9 10 11 12 13
| class Fu{ public final void show(){ sout('父类的show方法'); } } class Zi extends Fu{ @override public void show(){ sout('子类的show方法'); } }
final int a = 10;
|
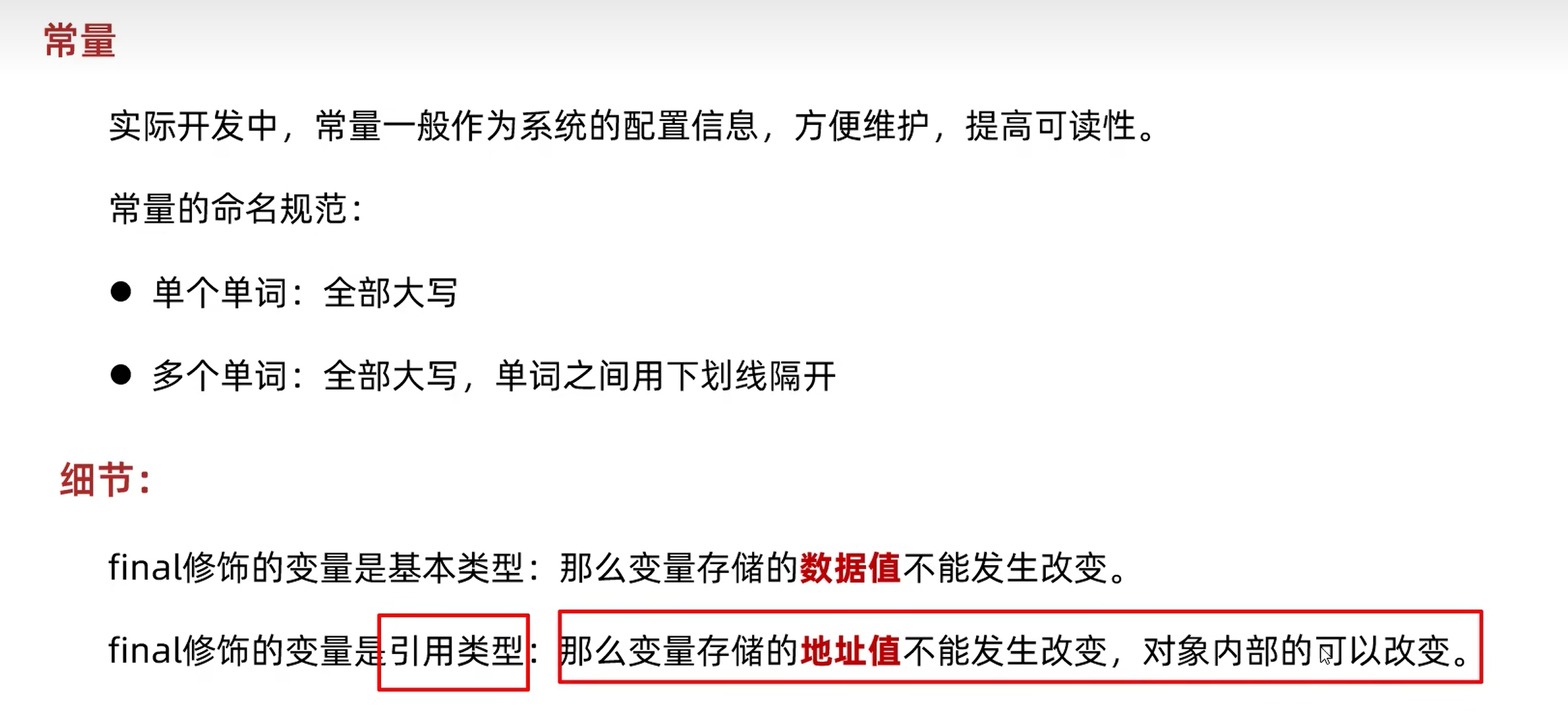
权限修饰符
- 权限修饰符:是用来控制一个成员能够被访问的范围的。
- 可以修饰成员变量,方法,构造方法,内部类。
1 2 3 4
| public class Student { private String name; private int age; }
|
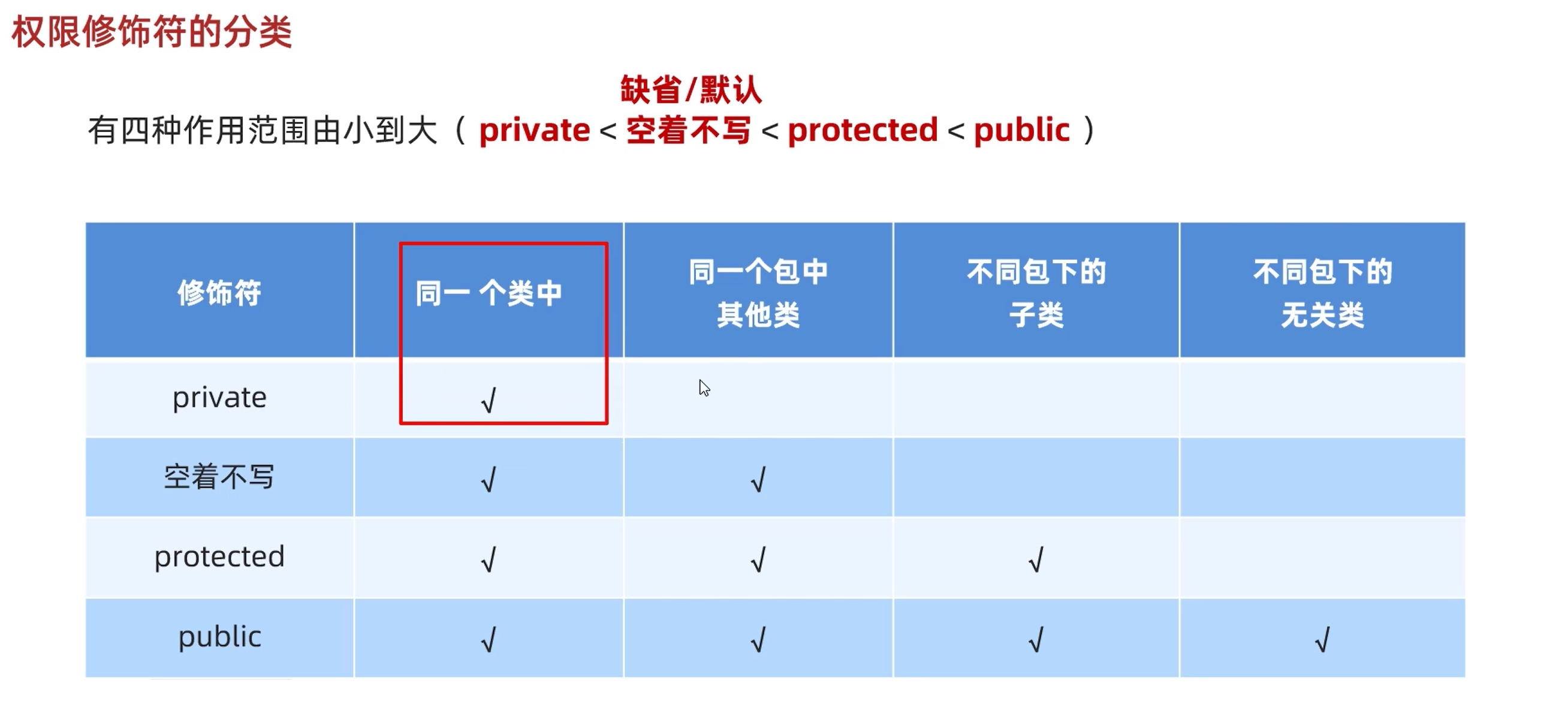
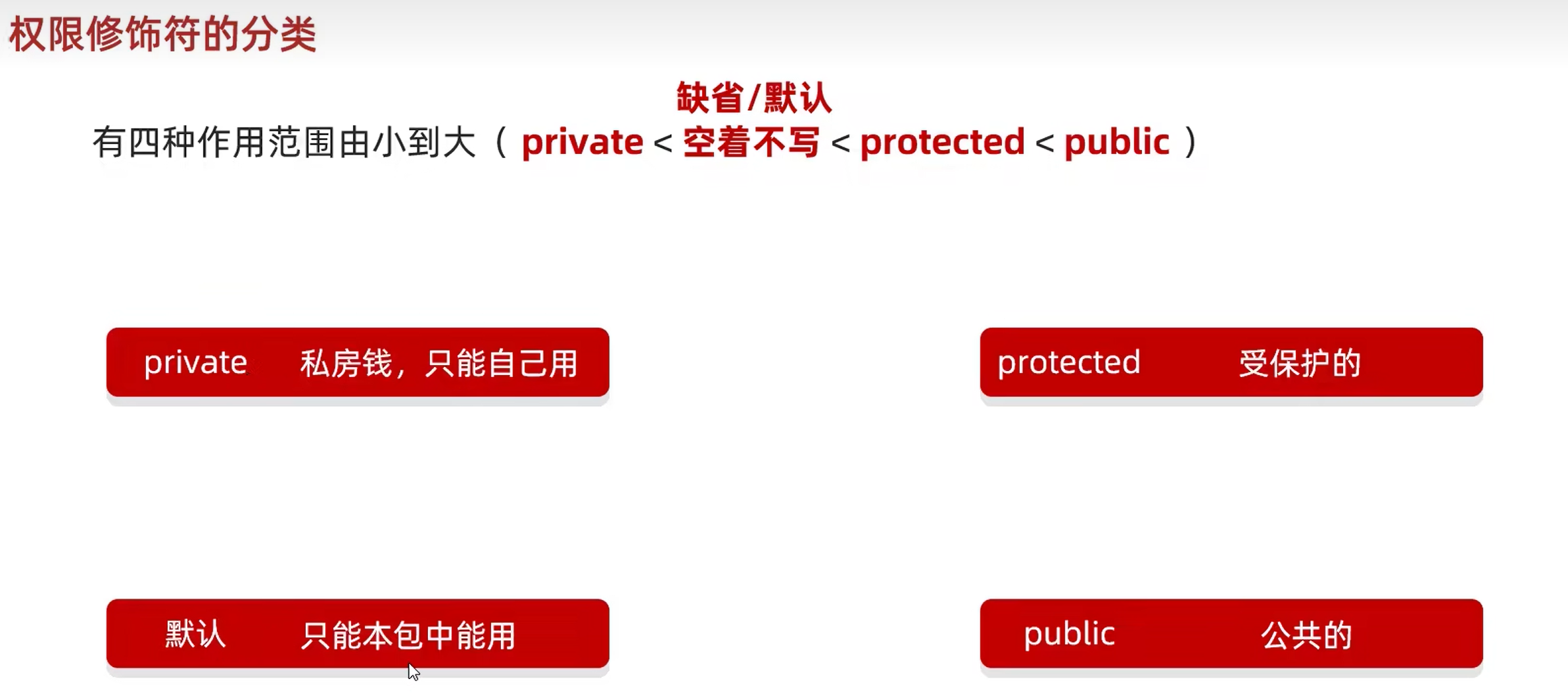
P134 代码块未看
Static
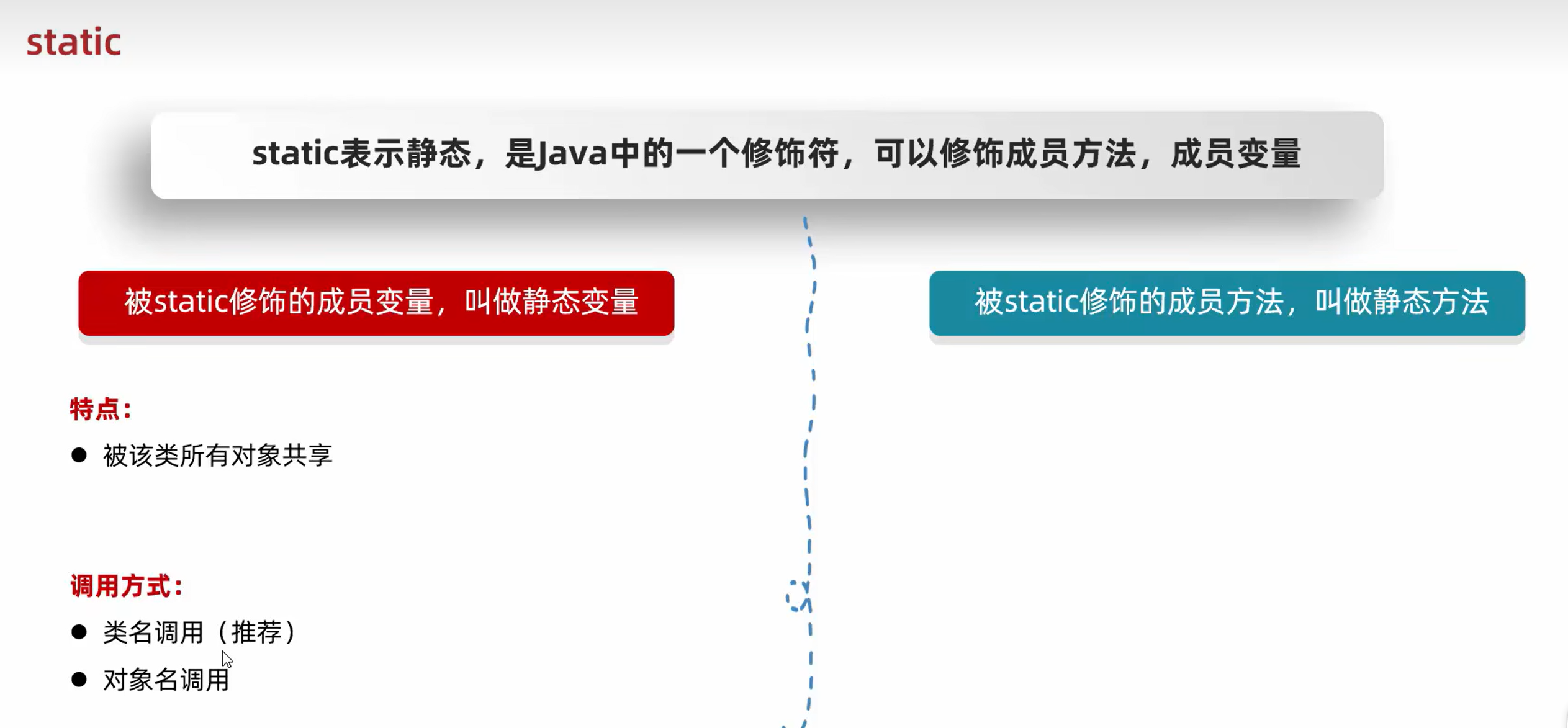
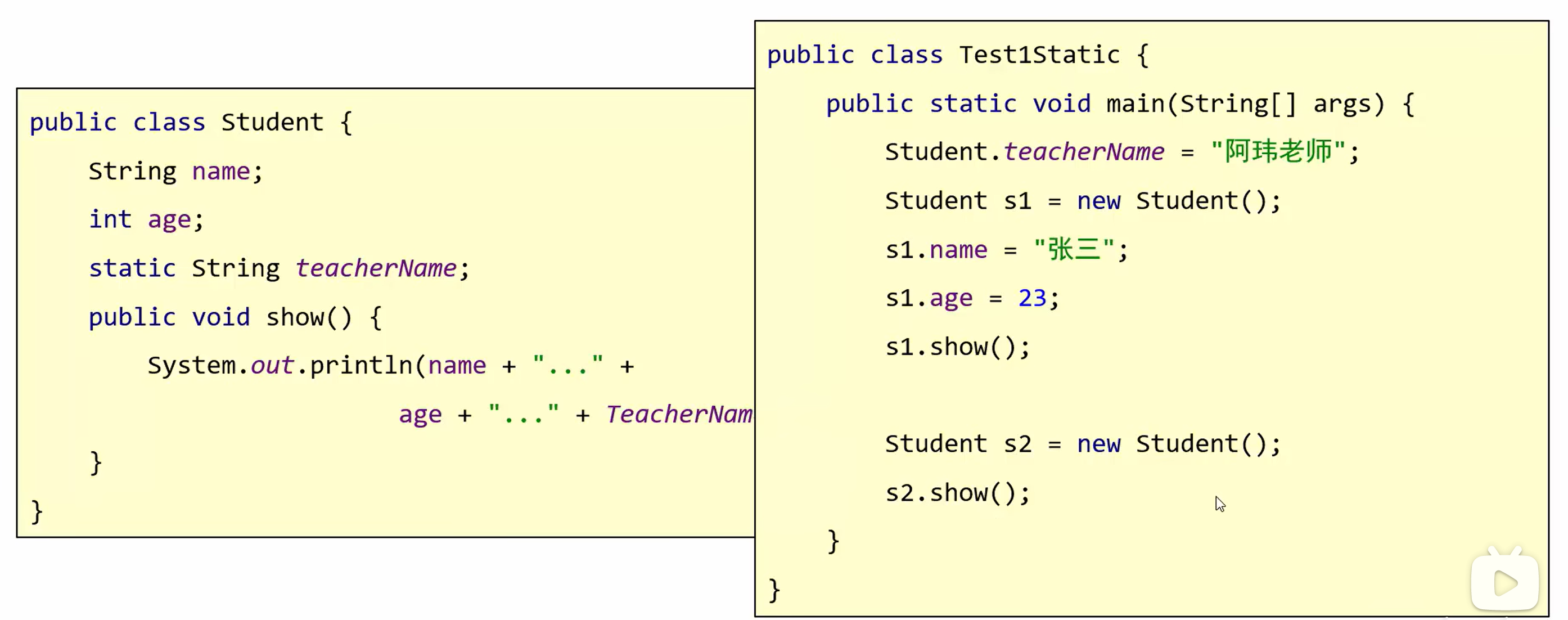
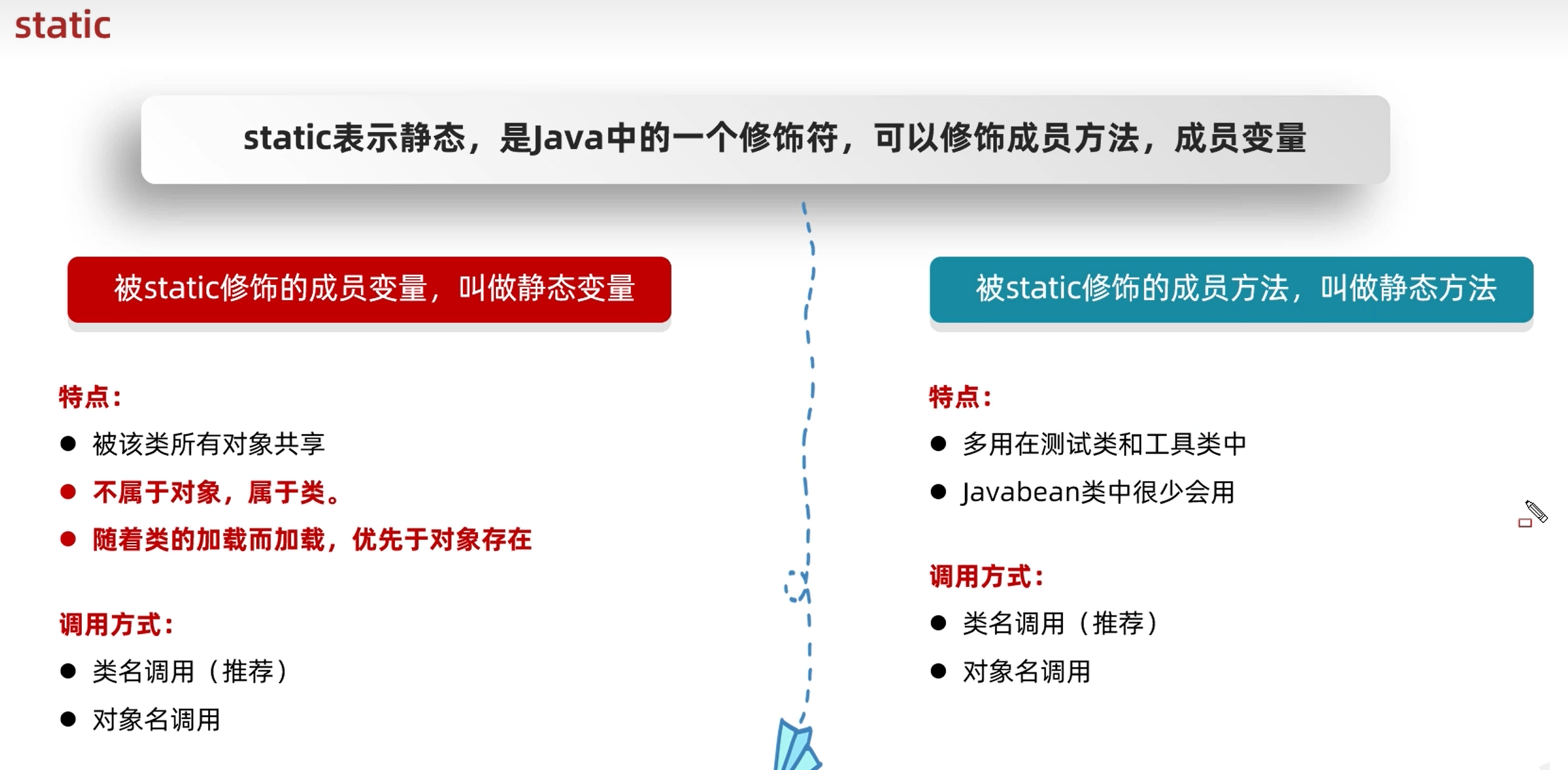
帮助我们做一些事情的,但是不描述任何事物的类。
用来描述一类事物的类,比如,student,teacher,dog,cat等。
用来检查其他类是否书写正确,带有main方法的类,是程序的入口。
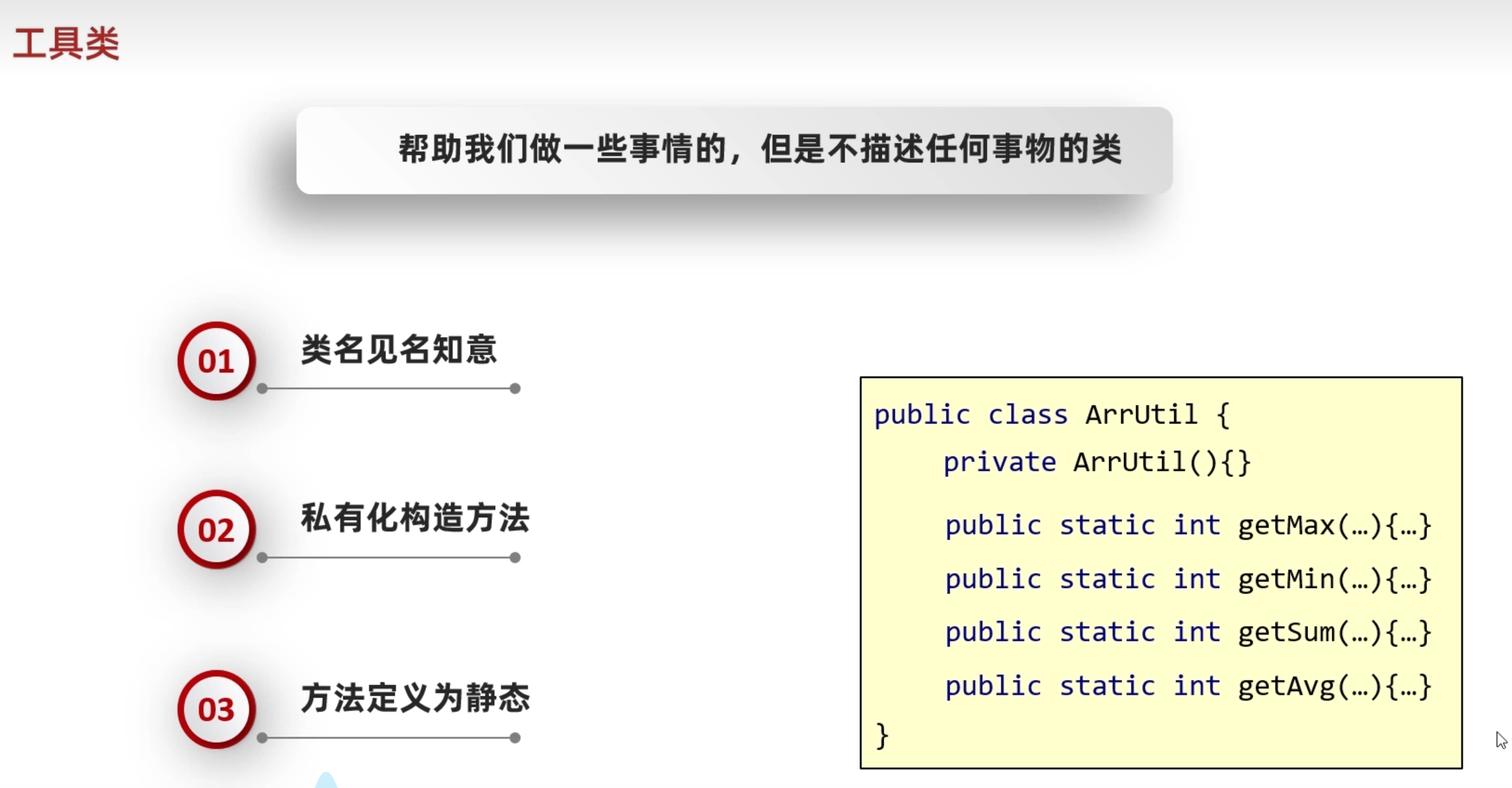
1 2 3 4 5 6 7 8 9
| public class ArrayUtil { private ArrayUtil(){ } public static String xxxxxx }
String ??? = ArrayUtil.方法名(参数)
|
P123未看
P135 抽象类
接口
为什么要存在接口?
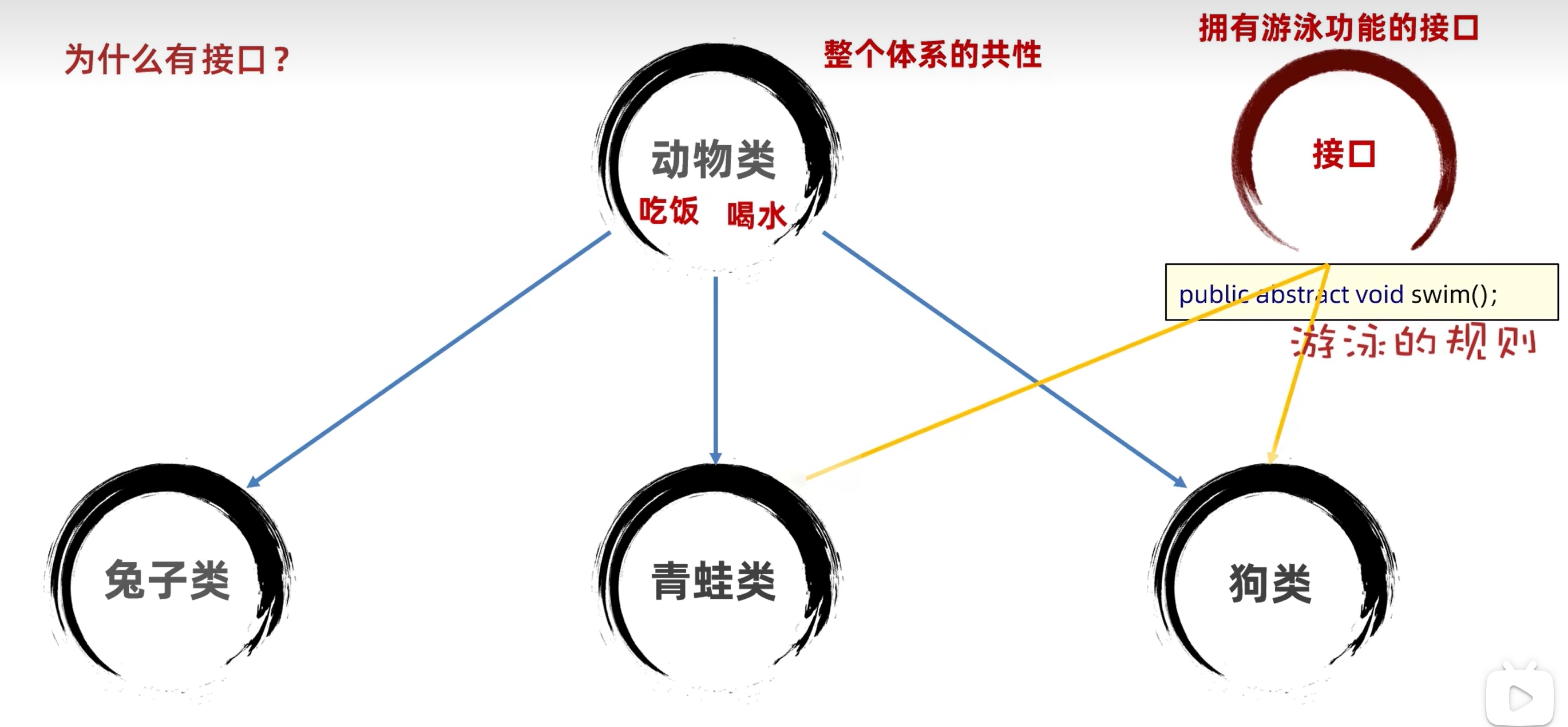
接口是一种规则,是对行为的抽象。
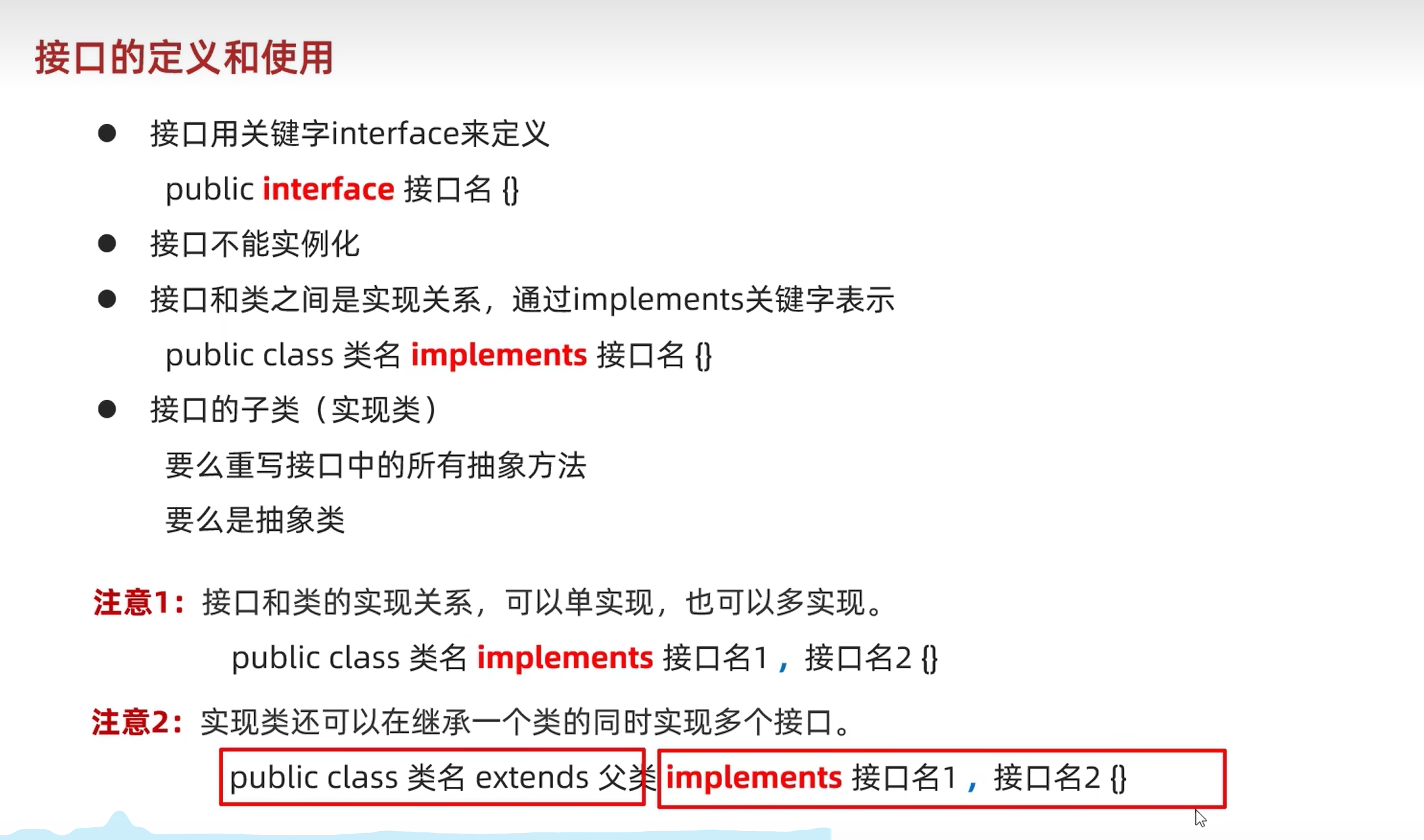
1 2 3
| public class Frog extends Animal implements Swim{ @override }
|
接口中成员的特点:
只能是常量
默认修饰符: public static final
没有
只能是抽象方法
默认修饰符: public abstract
接口和类之间的关系
继承关系,只能单继承不能多继承,但可以多层继承。
实现关系,可以但实现,也可以多实现,还可以在继承一个类的同时实现多个接口。
继承关系,可以单继承也可以多继承。
内部类
类的五大成员:
属性,方法,构造方法,代码块,内部类。
在一个类的里面,再定义一个类。
e.g:
1 2 3 4 5
| public class Outer { public class Inner{ } }
|
为什么要学习内部类?
需求:写一个javabean类描述汽车。
属性:品牌,车龄,颜色,发动机品牌,使用年限。
1 2 3 4 5 6 7 8
| public class Car { String carName; int carAge; String engineName; int engineAge; ......... }
|
单实际上,发动机算是个独立的个体:
1 2 3 4 5 6 7 8 9 10
| public class Car { String carName; int carAge; ......... }
public class Engine{ String engineName; int engineAge; }
|
但实际上发动机这东西要依赖车存在,所以可以依靠内部类实现:
1 2 3 4 5 6 7 8 9
| public class Car { String carName; int carAge; class Engine{ String engineName; int engineAge; } }
|
内部类的访问特点:
- 内部类可以直接访问外部类的成员,包括私有
- 外部类要访问内部类的成员,必须创建对象
P192 泛型
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| import org.apache.commons.collections.Transformer; import org.apache.commons.collections.functors.InvokerTransformer; import org.omg.SendingContext.RunTime; import java.lang.reflect.Method;
public class Test02 { public static void main(String[] args) throws Exception { Method getRuntimeMethod = (Method) new InvokerTransformer("getMethod", new Class[]{String.class, Class[].class}, new Object[]{"getRuntime", null}).transform(Runtime.class); Runtime r = (Runtime) new InvokerTransformer("invoke", new Class[]{Object.class, Object[].class}, new Object[]{null, null}).transform(getRuntimeMethod);
new InvokerTransformer("exec", new Class[]{String.class}, new Obj ect[]{"calc"}).transform(r); } }
|